Merge branch 'dev'
commit
9359125120
|
@ -0,0 +1,42 @@
|
|||
from inkplate2 import Inkplate
|
||||
from soldered_logo import *
|
||||
import time
|
||||
|
||||
display = Inkplate()
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using the display, like in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
|
||||
# Print some text
|
||||
display.printText(5, 8, "Welcome to Inkplate 2")
|
||||
|
||||
# Print some larger text in red
|
||||
display.setTextSize(2)
|
||||
display.printText(5, 20, "MicroPython!", display.RED)
|
||||
|
||||
# Fill a black circle and draw some white and red circles in it
|
||||
display.fillCircle(178, 16, 15, display.BLACK)
|
||||
display.drawCircle(178, 16, 13, display.RED)
|
||||
display.drawCircle(178, 16, 9, display.WHITE)
|
||||
display.drawCircle(178, 16, 4, display.RED)
|
||||
|
||||
# Draw a red checkerboard pattern
|
||||
for x in range(30):
|
||||
display.fillRect(0 + (5*x*2), 38, 5, 5, display.RED)
|
||||
|
||||
for x in range(30):
|
||||
display.fillRect(5 + (5*x*2), 42, 5, 5, display.RED)
|
||||
|
||||
# Draw some lines
|
||||
display.drawLine(0, 49, 214, 49, display.BLACK)
|
||||
display.drawLine(0, 51, 214, 51, display.RED)
|
||||
display.drawLine(0, 53, 214, 53, display.BLACK)
|
||||
display.drawLine(0, 55, 214, 55, display.RED)
|
||||
|
||||
# Draw a bitmap image
|
||||
display.drawBitmap(0, 58, soldered_logo, 211, 44, display.RED)
|
||||
|
||||
# Display everything on the ePaper - must be called!
|
||||
display.display()
|
|
@ -0,0 +1,64 @@
|
|||
import network
|
||||
import time
|
||||
from inkplate2 import Inkplate
|
||||
|
||||
ssid = ""
|
||||
password = ""
|
||||
|
||||
# Function which connects to WiFi
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_basics.html
|
||||
def do_connect():
|
||||
import network
|
||||
|
||||
# Connect to WiFi
|
||||
sta_if = network.WLAN(network.STA_IF)
|
||||
if not sta_if.isconnected():
|
||||
print("connecting to network...")
|
||||
sta_if.active(True)
|
||||
sta_if.connect(ssid, password)
|
||||
while not sta_if.isconnected():
|
||||
pass
|
||||
print("network config:", sta_if.ifconfig())
|
||||
|
||||
# Does a HTTP GET request
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_tcp.html
|
||||
def http_get(url):
|
||||
import socket
|
||||
res = ""
|
||||
_, _, host, path = url.split("/", 3)
|
||||
addr = socket.getaddrinfo(host, 80)[0][-1]
|
||||
s = socket.socket()
|
||||
s.connect(addr)
|
||||
s.send(bytes("GET /%s HTTP/1.0\r\nHost: %s\r\n\r\n" % (path, host), "utf8"))
|
||||
while True:
|
||||
data = s.recv(100)
|
||||
if data:
|
||||
res += str(data, "utf8")
|
||||
else:
|
||||
break
|
||||
s.close()
|
||||
|
||||
return res
|
||||
|
||||
|
||||
# Calling functions defined above
|
||||
do_connect()
|
||||
|
||||
# Do a GET request to the micropython test page
|
||||
# If you were to do a GET request to a different page, change the URL here
|
||||
response = http_get("http://micropython.org/ks/test.html")
|
||||
|
||||
# Initialise our Inkplate object
|
||||
display = Inkplate()
|
||||
display.begin()
|
||||
|
||||
# Print the GET response in lines
|
||||
cnt = 0
|
||||
for x in response.split("\n"):
|
||||
display.printText(
|
||||
10, 10 + cnt, x.upper()
|
||||
) # Default font has only upper case letters
|
||||
cnt += 10
|
||||
|
||||
# Display image from buffer
|
||||
display.display()
|
|
@ -0,0 +1,41 @@
|
|||
from inkplate5 import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, like in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
for r in range(4):
|
||||
# Sets the screen rotation
|
||||
display.setRotation(r)
|
||||
|
||||
# All drawing functions
|
||||
display.drawPixel(100, 100, display.BLACK)
|
||||
display.drawRect(50, 50, 75, 75, display.BLACK)
|
||||
display.drawCircle(200, 200, 30, display.BLACK)
|
||||
display.fillCircle(300, 300, 30, display.BLACK)
|
||||
display.drawFastHLine(20, 100, 50, display.BLACK)
|
||||
display.drawFastVLine(100, 20, 50, display.BLACK)
|
||||
display.drawLine(100, 100, 400, 400, display.BLACK)
|
||||
display.drawRoundRect(100, 10, 100, 100, 10, display.BLACK)
|
||||
display.fillRoundRect(10, 100, 100, 100, 10, display.BLACK)
|
||||
display.drawTriangle(300, 100, 400, 150, 400, 100, display.BLACK)
|
||||
|
||||
print("rotation "+str(r))
|
||||
|
||||
if display.rotation % 2 == 0:
|
||||
display.fillTriangle(500, 101, 400, 150, 400, 100, display.BLACK)
|
||||
display.display()
|
||||
time.sleep(5)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
display.drawBitmap(250, 420, image, 576, 100)
|
||||
|
||||
#Use display.partialUpdate instead of display.display() to draw only updated pixels
|
||||
display.partialUpdate()
|
|
@ -0,0 +1,33 @@
|
|||
# Include needed libraries
|
||||
|
||||
from inkplate5 import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
|
||||
# Initialize inkplate display
|
||||
display = Inkplate(Inkplate.INKPLATE_2BIT)
|
||||
|
||||
|
||||
# Main function, you can make infinite while loop inside this to run code indefinitely
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
# Draw palet of posible colors
|
||||
#use color values 0, 1, 2, 3
|
||||
display.writeFillRect(0, 0, 25, 600, 3)
|
||||
display.writeFillRect(25, 0, 25, 600, 2)
|
||||
display.writeFillRect(50, 0, 25, 600, 1)
|
||||
display.writeFillRect(75, 0, 25, 600, 0)
|
||||
|
||||
display.display()
|
||||
time.sleep(3)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
display.drawBitmap(120, 200, image, 576, 100)
|
||||
display.display()
|
||||
time.sleep(10)
|
|
@ -0,0 +1,22 @@
|
|||
from inkplate5 import Inkplate
|
||||
from image import *
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
if __name__ == "__main__":
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
battery = str(display.readBattery())
|
||||
|
||||
display.setTextSize(2)
|
||||
display.printText(100, 100, "batt: " + battery + "V")
|
||||
display.display()
|
||||
|
||||
|
||||
temperature = str(display.readTemperature())
|
||||
|
||||
display.setTextSize(2)
|
||||
display.printText(100, 150, "TEMP: " + temperature + "C")
|
||||
display.display()
|
|
@ -0,0 +1,60 @@
|
|||
import network
|
||||
import time
|
||||
from inkplate5 import Inkplate
|
||||
|
||||
ssid = ""
|
||||
password = ""
|
||||
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_basics.html
|
||||
def do_connect():
|
||||
import network
|
||||
|
||||
sta_if = network.WLAN(network.STA_IF)
|
||||
if not sta_if.isconnected():
|
||||
print("connecting to network...")
|
||||
sta_if.active(True)
|
||||
sta_if.connect(ssid, password)
|
||||
while not sta_if.isconnected():
|
||||
pass
|
||||
print("network config:", sta_if.ifconfig())
|
||||
|
||||
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_tcp.html
|
||||
def http_get(url):
|
||||
import socket
|
||||
|
||||
res = ""
|
||||
_, _, host, path = url.split("/", 3)
|
||||
addr = socket.getaddrinfo(host, 80)[0][-1]
|
||||
s = socket.socket()
|
||||
s.connect(addr)
|
||||
s.send(bytes("GET /%s HTTP/1.0\r\nHost: %s\r\n\r\n" % (path, host), "utf8"))
|
||||
while True:
|
||||
data = s.recv(100)
|
||||
if data:
|
||||
res += str(data, "utf8")
|
||||
else:
|
||||
break
|
||||
s.close()
|
||||
|
||||
return res
|
||||
|
||||
|
||||
# Calling functions defined above
|
||||
do_connect()
|
||||
response = http_get("http://micropython.org/ks/test.html")
|
||||
|
||||
# Initialise our Inkplate object
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
display.begin()
|
||||
display.setTextSize(2)
|
||||
# Print response in lines
|
||||
cnt = 0
|
||||
for x in response.split("\n"):
|
||||
display.printText(
|
||||
20, 20 + cnt, x.upper()
|
||||
) # Default font has only upper case letters
|
||||
cnt += 20
|
||||
|
||||
# Display image from buffer
|
||||
display.display()
|
|
@ -0,0 +1,21 @@
|
|||
import os, time
|
||||
from inkplate5 import Inkplate
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_2BIT)
|
||||
display.begin()
|
||||
|
||||
# This prints all the files on card
|
||||
print(os.listdir("/sd"))
|
||||
|
||||
f = open("sd/text.txt", "r")
|
||||
|
||||
# Print file contents
|
||||
print(f.read())
|
||||
f.close()
|
||||
|
||||
time.sleep(5)
|
||||
|
||||
# Utterly slow, can take minutes :(
|
||||
display.drawImageFile(0, 0, "sd/1.bmp")
|
||||
|
||||
display.display()
|
|
@ -28,8 +28,8 @@ if __name__ == "__main__":
|
|||
|
||||
if display.rotation % 2 == 0:
|
||||
display.fillTriangle(500, 101, 400, 150, 400, 100, display.BLACK)
|
||||
display.display()
|
||||
time.sleep(5)
|
||||
display.display()
|
||||
time.sleep(5)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
|
|
|
@ -5,6 +5,9 @@ from inkplate6_COLOR import Inkplate
|
|||
display = Inkplate()
|
||||
display.begin()
|
||||
|
||||
# SD Card must be initialised with this function
|
||||
display.initSDCard()
|
||||
|
||||
# This prints all the files on card
|
||||
print(os.listdir("/sd"))
|
||||
|
||||
|
@ -13,3 +16,14 @@ f = open("sd/text.txt", "r")
|
|||
# Print file contents
|
||||
print(f.read())
|
||||
f.close()
|
||||
|
||||
time.sleep(5)
|
||||
|
||||
display.drawImageFile(0, 0, "sd/1.bmp")
|
||||
|
||||
# You can turn off the power to the SD card to save power
|
||||
display.SDCardSleep()
|
||||
# To turn it back on, use:
|
||||
# display.SDCardWake()
|
||||
|
||||
display.display()
|
||||
|
|
|
@ -0,0 +1,61 @@
|
|||
import time
|
||||
from PCAL6416A import *
|
||||
|
||||
from inkplate6_COLOR import Inkplate
|
||||
|
||||
display = Inkplate()
|
||||
|
||||
# This script demonstrates using all the available GPIO expander pins as output
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
|
||||
# pin = display.gpioExpanderPin(pin,mode)
|
||||
# Supported modes: modeINPUT, modeINPUT_PULLUP, modeINPUT_PULLDOWN, modeOUTPUT
|
||||
# Supported pins on Inkplate 6 COLOR are listed below
|
||||
|
||||
expander_P0_0 = display.gpioExpanderPin(0, modeOUTPUT)
|
||||
expander_P0_1 = display.gpioExpanderPin(1, modeOUTPUT)
|
||||
expander_P0_2 = display.gpioExpanderPin(2, modeOUTPUT)
|
||||
expander_P0_3 = display.gpioExpanderPin(3, modeOUTPUT)
|
||||
expander_P0_4 = display.gpioExpanderPin(4, modeOUTPUT)
|
||||
expander_P0_5 = display.gpioExpanderPin(5, modeOUTPUT)
|
||||
expander_P0_6 = display.gpioExpanderPin(6, modeOUTPUT)
|
||||
expander_P0_7 = display.gpioExpanderPin(7, modeOUTPUT)
|
||||
|
||||
expander_P1_0 = display.gpioExpanderPin(8, modeOUTPUT)
|
||||
expander_P1_1 = display.gpioExpanderPin(9, modeOUTPUT)
|
||||
expander_P1_2 = display.gpioExpanderPin(10, modeOUTPUT)
|
||||
expander_P1_3 = display.gpioExpanderPin(11, modeOUTPUT)
|
||||
expander_P1_4 = display.gpioExpanderPin(12, modeOUTPUT)
|
||||
expander_P1_5 = display.gpioExpanderPin(13, modeOUTPUT)
|
||||
expander_P1_6 = display.gpioExpanderPin(14, modeOUTPUT)
|
||||
expander_P1_7 = display.gpioExpanderPin(15, modeOUTPUT)
|
||||
|
||||
pins = (expander_P0_0,
|
||||
expander_P0_1,
|
||||
expander_P0_2,
|
||||
expander_P0_3,
|
||||
expander_P0_4,
|
||||
expander_P0_5,
|
||||
expander_P0_6,
|
||||
expander_P0_7,
|
||||
expander_P1_0,
|
||||
expander_P1_1,
|
||||
expander_P1_2,
|
||||
expander_P1_3,
|
||||
expander_P1_4,
|
||||
expander_P1_5,
|
||||
expander_P1_6,
|
||||
expander_P1_7,
|
||||
)
|
||||
|
||||
# This example writes a 0.2s pulse on the pins consecutively to test the output
|
||||
|
||||
while (1):
|
||||
for pin in pins:
|
||||
pin.digitalWrite(1)
|
||||
time.sleep(0.2)
|
||||
pin.digitalWrite(0)
|
||||
time.sleep(0.2)
|
|
@ -0,0 +1,39 @@
|
|||
from soldered_inkplate10 import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
for r in range(4):
|
||||
# Sets the screen rotation
|
||||
display.setRotation(r)
|
||||
|
||||
# All drawing functions
|
||||
display.drawPixel(100, 100, display.BLACK)
|
||||
display.drawRect(50, 50, 75, 75, display.BLACK)
|
||||
display.drawCircle(200, 200, 30, display.BLACK)
|
||||
display.fillCircle(300, 300, 30, display.BLACK)
|
||||
display.drawFastHLine(20, 100, 50, display.BLACK)
|
||||
display.drawFastVLine(100, 20, 50, display.BLACK)
|
||||
display.drawLine(100, 100, 400, 400, display.BLACK)
|
||||
display.drawRoundRect(100, 10, 100, 100, 10, display.BLACK)
|
||||
display.fillRoundRect(10, 100, 100, 100, 10, display.BLACK)
|
||||
display.drawTriangle(300, 100, 400, 150, 400, 100, display.BLACK)
|
||||
|
||||
if display.rotation % 2 == 0:
|
||||
display.fillTriangle(500, 101, 400, 150, 400, 100, display.BLACK)
|
||||
display.display()
|
||||
time.sleep(5)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
display.drawBitmap(120, 200, image, 576, 100)
|
||||
|
||||
#Use display.partialUpdate instead of display.display() to draw only updated pixels
|
||||
display.partialUpdate()
|
|
@ -0,0 +1,32 @@
|
|||
# Include needed libraries
|
||||
|
||||
from soldered_inkplate10 import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
# Initialize inkplate display
|
||||
display = Inkplate(Inkplate.INKPLATE_2BIT)
|
||||
|
||||
|
||||
# Main function, you can make infinite while loop inside this to run code indefinitely
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
# Draw palet of posible colors
|
||||
#use color values 0, 1, 2, 3
|
||||
display.writeFillRect(0, 0, 25, 600, 3)
|
||||
display.writeFillRect(25, 0, 25, 600, 2)
|
||||
display.writeFillRect(50, 0, 25, 600, 1)
|
||||
display.writeFillRect(75, 0, 25, 600, 0)
|
||||
|
||||
display.display()
|
||||
time.sleep(3)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
display.drawBitmap(120, 200, image, 576, 100)
|
||||
display.display()
|
||||
time.sleep(10)
|
|
@ -0,0 +1,22 @@
|
|||
from soldered_inkplate10 import Inkplate
|
||||
from image import *
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
if __name__ == "__main__":
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
battery = str(display.readBattery())
|
||||
|
||||
display.setTextSize(2)
|
||||
display.printText(100, 100, "batt: " + battery + "V")
|
||||
display.display()
|
||||
|
||||
|
||||
temperature = str(display.readTemperature())
|
||||
|
||||
display.setTextSize(2)
|
||||
display.printText(100, 150, "TEMP: " + temperature + "C")
|
||||
display.display()
|
|
@ -0,0 +1,60 @@
|
|||
import network
|
||||
import time
|
||||
from soldered_inkplate10 import Inkplate
|
||||
|
||||
ssid = "e-radionica.com"
|
||||
password = "croduino"
|
||||
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_basics.html
|
||||
def do_connect():
|
||||
import network
|
||||
|
||||
sta_if = network.WLAN(network.STA_IF)
|
||||
if not sta_if.isconnected():
|
||||
print("connecting to network...")
|
||||
sta_if.active(True)
|
||||
sta_if.connect(ssid, password)
|
||||
while not sta_if.isconnected():
|
||||
pass
|
||||
print("network config:", sta_if.ifconfig())
|
||||
|
||||
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_tcp.html
|
||||
def http_get(url):
|
||||
import socket
|
||||
|
||||
res = ""
|
||||
_, _, host, path = url.split("/", 3)
|
||||
addr = socket.getaddrinfo(host, 80)[0][-1]
|
||||
s = socket.socket()
|
||||
s.connect(addr)
|
||||
s.send(bytes("GET /%s HTTP/1.0\r\nHost: %s\r\n\r\n" % (path, host), "utf8"))
|
||||
while True:
|
||||
data = s.recv(100)
|
||||
if data:
|
||||
res += str(data, "utf8")
|
||||
else:
|
||||
break
|
||||
s.close()
|
||||
|
||||
return res
|
||||
|
||||
|
||||
# Calling functions defined above
|
||||
do_connect()
|
||||
response = http_get("http://micropython.org/ks/test.html")
|
||||
|
||||
# Initialise our Inkplate object
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
display.begin()
|
||||
|
||||
# Print response in lines
|
||||
cnt = 0
|
||||
for x in response.split("\n"):
|
||||
display.printText(
|
||||
10, 10 + cnt, x.upper()
|
||||
) # Default font has only upper case letters
|
||||
cnt += 10
|
||||
|
||||
# Display image from buffer
|
||||
display.display()
|
|
@ -0,0 +1,28 @@
|
|||
import os, time
|
||||
from soldered_inkplate10 import Inkplate
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_2BIT)
|
||||
display.begin()
|
||||
|
||||
# SD Card must be initialised with this function
|
||||
display.initSDCard()
|
||||
|
||||
# This prints all the files on card
|
||||
print(os.listdir("/sd"))
|
||||
|
||||
f = open("sd/text.txt", "r")
|
||||
|
||||
# Print file contents
|
||||
print(f.read())
|
||||
f.close()
|
||||
|
||||
time.sleep(5)
|
||||
|
||||
display.drawImageFile(0, 0, "sd/1.bmp")
|
||||
|
||||
# You can turn off the power to the SD card to save power
|
||||
display.SDCardSleep()
|
||||
# To turn it back on, use:
|
||||
# display.SDCardWake()
|
||||
|
||||
display.display()
|
|
@ -0,0 +1,73 @@
|
|||
import time
|
||||
from PCAL6416A import *
|
||||
|
||||
from soldered_inkplate10 import Inkplate
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
# This script demonstrates using all the available GPIO expander pins as output
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
|
||||
# pin = display.gpioExpanderPin(gpioExpander,pin,mode)
|
||||
# Supported gpio expanders on Soldered Inkplate 10: 1, 2
|
||||
# Supported modes: modeINPUT, modeINPUT_PULLUP, modeINPUT_PULLDOWN, modeOUTPUT
|
||||
# Supported pins on Soldered Inkplate 10 are listed below
|
||||
|
||||
expander1_P1_3 = display.gpioExpanderPin(1, 11, modeOUTPUT)
|
||||
expander1_P1_4 = display.gpioExpanderPin(1, 12, modeOUTPUT)
|
||||
expander1_P1_5 = display.gpioExpanderPin(1, 13, modeOUTPUT)
|
||||
expander1_P1_6 = display.gpioExpanderPin(1, 14, modeOUTPUT)
|
||||
expander1_P1_7 = display.gpioExpanderPin(1, 15, modeOUTPUT)
|
||||
|
||||
expander2_P0_0 = display.gpioExpanderPin(2, 0, modeOUTPUT)
|
||||
expander2_P0_1 = display.gpioExpanderPin(2, 1, modeOUTPUT)
|
||||
expander2_P0_2 = display.gpioExpanderPin(2, 2, modeOUTPUT)
|
||||
expander2_P0_3 = display.gpioExpanderPin(2, 3, modeOUTPUT)
|
||||
expander2_P0_4 = display.gpioExpanderPin(2, 4, modeOUTPUT)
|
||||
expander2_P0_5 = display.gpioExpanderPin(2, 5, modeOUTPUT)
|
||||
expander2_P0_6 = display.gpioExpanderPin(2, 6, modeOUTPUT)
|
||||
expander2_P0_7 = display.gpioExpanderPin(2, 7, modeOUTPUT)
|
||||
|
||||
expander2_P1_0 = display.gpioExpanderPin(2, 8, modeOUTPUT)
|
||||
expander2_P1_1 = display.gpioExpanderPin(2, 9, modeOUTPUT)
|
||||
expander2_P1_2 = display.gpioExpanderPin(2, 10, modeOUTPUT)
|
||||
expander2_P1_3 = display.gpioExpanderPin(2, 11, modeOUTPUT)
|
||||
expander2_P1_4 = display.gpioExpanderPin(2, 12, modeOUTPUT)
|
||||
expander2_P1_5 = display.gpioExpanderPin(2, 13, modeOUTPUT)
|
||||
expander2_P1_6 = display.gpioExpanderPin(2, 14, modeOUTPUT)
|
||||
expander2_P1_7 = display.gpioExpanderPin(2, 15, modeOUTPUT)
|
||||
|
||||
pins = (expander1_P1_3,
|
||||
expander1_P1_4,
|
||||
expander1_P1_5,
|
||||
expander1_P1_6,
|
||||
expander1_P1_7,
|
||||
expander2_P0_0,
|
||||
expander2_P0_1,
|
||||
expander2_P0_2,
|
||||
expander2_P0_3,
|
||||
expander2_P0_4,
|
||||
expander2_P0_5,
|
||||
expander2_P0_6,
|
||||
expander2_P0_7,
|
||||
expander2_P1_0,
|
||||
expander2_P1_1,
|
||||
expander2_P1_2,
|
||||
expander2_P1_3,
|
||||
expander2_P1_4,
|
||||
expander2_P1_5,
|
||||
expander2_P1_6,
|
||||
expander2_P1_7,
|
||||
)
|
||||
|
||||
# This example writes a 0.2s pulse on the pins consecutively to test the output
|
||||
|
||||
while (1):
|
||||
for pin in pins:
|
||||
pin.digitalWrite(1)
|
||||
time.sleep(0.2)
|
||||
pin.digitalWrite(0)
|
||||
time.sleep(0.2)
|
|
@ -0,0 +1,39 @@
|
|||
from soldered_inkplate6 import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
for r in range(4):
|
||||
# Sets the screen rotation
|
||||
display.setRotation(r)
|
||||
|
||||
# All drawing functions
|
||||
display.drawPixel(100, 100, display.BLACK)
|
||||
display.drawRect(50, 50, 75, 75, display.BLACK)
|
||||
display.drawCircle(200, 200, 30, display.BLACK)
|
||||
display.fillCircle(300, 300, 30, display.BLACK)
|
||||
display.drawFastHLine(20, 100, 50, display.BLACK)
|
||||
display.drawFastVLine(100, 20, 50, display.BLACK)
|
||||
display.drawLine(100, 100, 400, 400, display.BLACK)
|
||||
display.drawRoundRect(100, 10, 100, 100, 10, display.BLACK)
|
||||
display.fillRoundRect(10, 100, 100, 100, 10, display.BLACK)
|
||||
display.drawTriangle(300, 100, 400, 150, 400, 100, display.BLACK)
|
||||
|
||||
if display.rotation % 2 == 0:
|
||||
display.fillTriangle(500, 101, 400, 150, 400, 100, display.BLACK)
|
||||
display.display()
|
||||
time.sleep(5)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
display.drawBitmap(120, 200, image, 576, 100)
|
||||
|
||||
#Use display.partialUpdate instead of display.display() to draw only updated pixels
|
||||
display.partialUpdate()
|
|
@ -0,0 +1,33 @@
|
|||
# Include needed libraries
|
||||
|
||||
from soldered_inkplate6 import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
|
||||
# Initialize inkplate display
|
||||
display = Inkplate(Inkplate.INKPLATE_2BIT)
|
||||
|
||||
|
||||
# Main function, you can make infinite while loop inside this to run code indefinitely
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
# Draw palet of posible colors
|
||||
#use color values 0, 1, 2, 3
|
||||
display.writeFillRect(0, 0, 25, 600, 3)
|
||||
display.writeFillRect(25, 0, 25, 600, 2)
|
||||
display.writeFillRect(50, 0, 25, 600, 1)
|
||||
display.writeFillRect(75, 0, 25, 600, 0)
|
||||
|
||||
display.display()
|
||||
time.sleep(3)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
display.drawBitmap(120, 200, image, 576, 100)
|
||||
display.display()
|
||||
time.sleep(10)
|
|
@ -0,0 +1,22 @@
|
|||
from soldered_inkplate6 import Inkplate
|
||||
from image import *
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
if __name__ == "__main__":
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
battery = str(display.readBattery())
|
||||
|
||||
display.setTextSize(2)
|
||||
display.printText(100, 100, "batt: " + battery + "V")
|
||||
display.display()
|
||||
|
||||
|
||||
temperature = str(display.readTemperature())
|
||||
|
||||
display.setTextSize(2)
|
||||
display.printText(100, 150, "TEMP: " + temperature + "C")
|
||||
display.display()
|
|
@ -0,0 +1,60 @@
|
|||
import network
|
||||
import time
|
||||
from soldered_inkplate6 import Inkplate
|
||||
|
||||
ssid = ""
|
||||
password = ""
|
||||
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_basics.html
|
||||
def do_connect():
|
||||
import network
|
||||
|
||||
sta_if = network.WLAN(network.STA_IF)
|
||||
if not sta_if.isconnected():
|
||||
print("connecting to network...")
|
||||
sta_if.active(True)
|
||||
sta_if.connect(ssid, password)
|
||||
while not sta_if.isconnected():
|
||||
pass
|
||||
print("network config:", sta_if.ifconfig())
|
||||
|
||||
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_tcp.html
|
||||
def http_get(url):
|
||||
import socket
|
||||
|
||||
res = ""
|
||||
_, _, host, path = url.split("/", 3)
|
||||
addr = socket.getaddrinfo(host, 80)[0][-1]
|
||||
s = socket.socket()
|
||||
s.connect(addr)
|
||||
s.send(bytes("GET /%s HTTP/1.0\r\nHost: %s\r\n\r\n" % (path, host), "utf8"))
|
||||
while True:
|
||||
data = s.recv(100)
|
||||
if data:
|
||||
res += str(data, "utf8")
|
||||
else:
|
||||
break
|
||||
s.close()
|
||||
|
||||
return res
|
||||
|
||||
|
||||
# Calling functions defined above
|
||||
do_connect()
|
||||
response = http_get("http://micropython.org/ks/test.html")
|
||||
|
||||
# Initialise our Inkplate object
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
display.begin()
|
||||
|
||||
# Print response in lines
|
||||
cnt = 0
|
||||
for x in response.split("\n"):
|
||||
display.printText(
|
||||
10, 10 + cnt, x.upper()
|
||||
) # Default font has only upper case letters
|
||||
cnt += 10
|
||||
|
||||
# Display image from buffer
|
||||
display.display()
|
|
@ -0,0 +1,28 @@
|
|||
import os, time
|
||||
from soldered_inkplate6 import Inkplate
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_2BIT)
|
||||
display.begin()
|
||||
|
||||
# SD Card must be initialised with this function
|
||||
display.initSDCard()
|
||||
|
||||
# This prints all the files on card
|
||||
print(os.listdir("/sd"))
|
||||
|
||||
f = open("sd/text.txt", "r")
|
||||
|
||||
# Print file contents
|
||||
print(f.read())
|
||||
f.close()
|
||||
|
||||
time.sleep(5)
|
||||
|
||||
display.drawImageFile(0, 0, "sd/1.bmp")
|
||||
|
||||
# You can turn off the power to the SD card to save power
|
||||
display.SDCardSleep()
|
||||
# To turn it back on, use:
|
||||
# display.SDCardWake()
|
||||
|
||||
display.display()
|
|
@ -0,0 +1,76 @@
|
|||
import time
|
||||
from PCAL6416A import *
|
||||
|
||||
from soldered_inkplate6 import Inkplate
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
# This script demonstrates using all the available GPIO expander pins as output
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
|
||||
# pin = display.gpioExpanderPin(gpioExpander,pin,mode)
|
||||
# Supported gpio expanders on Soldered Inkplate 10: 1, 2
|
||||
# Supported modes: modeINPUT, modeINPUT_PULLUP, modeINPUT_PULLDOWN, modeOUTPUT
|
||||
# Supported pins on Soldered Inkplate 10 are listed below
|
||||
|
||||
expander1_P1_1 = display.gpioExpanderPin(1, 9, modeOUTPUT)
|
||||
expander1_P1_2 = display.gpioExpanderPin(1, 10, modeOUTPUT)
|
||||
expander1_P1_3 = display.gpioExpanderPin(1, 11, modeOUTPUT)
|
||||
expander1_P1_4 = display.gpioExpanderPin(1, 12, modeOUTPUT)
|
||||
expander1_P1_5 = display.gpioExpanderPin(1, 13, modeOUTPUT)
|
||||
expander1_P1_6 = display.gpioExpanderPin(1, 14, modeOUTPUT)
|
||||
expander1_P1_7 = display.gpioExpanderPin(1, 15, modeOUTPUT)
|
||||
|
||||
expander2_P0_0 = display.gpioExpanderPin(2, 0, modeOUTPUT)
|
||||
expander2_P0_1 = display.gpioExpanderPin(2, 1, modeOUTPUT)
|
||||
expander2_P0_2 = display.gpioExpanderPin(2, 2, modeOUTPUT)
|
||||
expander2_P0_3 = display.gpioExpanderPin(2, 3, modeOUTPUT)
|
||||
expander2_P0_4 = display.gpioExpanderPin(2, 4, modeOUTPUT)
|
||||
expander2_P0_5 = display.gpioExpanderPin(2, 5, modeOUTPUT)
|
||||
expander2_P0_6 = display.gpioExpanderPin(2, 6, modeOUTPUT)
|
||||
expander2_P0_7 = display.gpioExpanderPin(2, 7, modeOUTPUT)
|
||||
|
||||
expander2_P1_0 = display.gpioExpanderPin(2, 8, modeOUTPUT)
|
||||
expander2_P1_1 = display.gpioExpanderPin(2, 9, modeOUTPUT)
|
||||
expander2_P1_2 = display.gpioExpanderPin(2, 10, modeOUTPUT)
|
||||
expander2_P1_3 = display.gpioExpanderPin(2, 11, modeOUTPUT)
|
||||
expander2_P1_4 = display.gpioExpanderPin(2, 12, modeOUTPUT)
|
||||
expander2_P1_5 = display.gpioExpanderPin(2, 13, modeOUTPUT)
|
||||
expander2_P1_6 = display.gpioExpanderPin(2, 14, modeOUTPUT)
|
||||
expander2_P1_7 = display.gpioExpanderPin(2, 15, modeOUTPUT)
|
||||
|
||||
pins = (expander1_P1_1,
|
||||
expander1_P1_2,
|
||||
expander1_P1_3,
|
||||
expander1_P1_5,
|
||||
expander1_P1_6,
|
||||
expander1_P1_7,
|
||||
expander2_P0_0,
|
||||
expander2_P0_1,
|
||||
expander2_P0_2,
|
||||
expander2_P0_3,
|
||||
expander2_P0_4,
|
||||
expander2_P0_5,
|
||||
expander2_P0_6,
|
||||
expander2_P0_7,
|
||||
expander2_P1_0,
|
||||
expander2_P1_1,
|
||||
expander2_P1_2,
|
||||
expander2_P1_3,
|
||||
expander2_P1_4,
|
||||
expander2_P1_5,
|
||||
expander2_P1_6,
|
||||
expander2_P1_7,
|
||||
)
|
||||
|
||||
# This example writes a 0.2s pulse on the pins consecutively to test the output
|
||||
|
||||
while (1):
|
||||
for pin in pins:
|
||||
pin.digitalWrite(1)
|
||||
time.sleep(0.2)
|
||||
pin.digitalWrite(0)
|
||||
time.sleep(0.2)
|
|
@ -0,0 +1,39 @@
|
|||
from soldered_inkplate6_PLUS import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
for r in range(4):
|
||||
# Sets the screen rotation
|
||||
display.setRotation(r)
|
||||
|
||||
# All drawing functions
|
||||
display.drawPixel(100, 100, display.BLACK)
|
||||
display.drawRect(50, 50, 75, 75, display.BLACK)
|
||||
display.drawCircle(200, 200, 30, display.BLACK)
|
||||
display.fillCircle(300, 300, 30, display.BLACK)
|
||||
display.drawFastHLine(20, 100, 50, display.BLACK)
|
||||
display.drawFastVLine(100, 20, 50, display.BLACK)
|
||||
display.drawLine(100, 100, 400, 400, display.BLACK)
|
||||
display.drawRoundRect(100, 10, 100, 100, 10, display.BLACK)
|
||||
display.fillRoundRect(10, 100, 100, 100, 10, display.BLACK)
|
||||
display.drawTriangle(300, 100, 400, 150, 400, 100, display.BLACK)
|
||||
|
||||
if display.rotation % 2 == 0:
|
||||
display.fillTriangle(500, 101, 400, 150, 400, 100, display.BLACK)
|
||||
display.display()
|
||||
time.sleep(5)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
display.drawBitmap(120, 200, image, 576, 100)
|
||||
|
||||
#Use display.partialUpdate instead of display.display() to draw only updated pixels
|
||||
display.partialUpdate()
|
|
@ -0,0 +1,33 @@
|
|||
# Include needed libraries
|
||||
|
||||
from soldered_inkplate6_PLUS import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
|
||||
# Initialize inkplate display
|
||||
display = Inkplate(Inkplate.INKPLATE_2BIT)
|
||||
|
||||
|
||||
# Main function, you can make infinite while loop inside this to run code indefinitely
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
# Draw palet of posible colors
|
||||
#use color values 0, 1, 2, 3
|
||||
display.writeFillRect(0, 0, 25, 600, 3)
|
||||
display.writeFillRect(25, 0, 25, 600, 2)
|
||||
display.writeFillRect(50, 0, 25, 600, 1)
|
||||
display.writeFillRect(75, 0, 25, 600, 0)
|
||||
|
||||
display.display()
|
||||
time.sleep(3)
|
||||
|
||||
# Draws image from bytearray
|
||||
display.setRotation(0)
|
||||
display.drawBitmap(120, 200, image, 576, 100)
|
||||
display.display()
|
||||
time.sleep(10)
|
|
@ -0,0 +1,22 @@
|
|||
from soldered_inkplate6_PLUS import Inkplate
|
||||
from image import *
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
if __name__ == "__main__":
|
||||
display.begin()
|
||||
display.clearDisplay()
|
||||
display.display()
|
||||
|
||||
battery = str(display.readBattery())
|
||||
|
||||
display.setTextSize(2)
|
||||
display.printText(100, 100, "batt: " + battery + "V")
|
||||
display.display()
|
||||
|
||||
|
||||
temperature = str(display.readTemperature())
|
||||
|
||||
display.setTextSize(2)
|
||||
display.printText(100, 150, "TEMP: " + temperature + "C")
|
||||
display.display()
|
|
@ -0,0 +1,60 @@
|
|||
import network
|
||||
import time
|
||||
from soldered_inkplate6_PLUS import Inkplate
|
||||
|
||||
ssid = ""
|
||||
password = ""
|
||||
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_basics.html
|
||||
def do_connect():
|
||||
import network
|
||||
|
||||
sta_if = network.WLAN(network.STA_IF)
|
||||
if not sta_if.isconnected():
|
||||
print("connecting to network...")
|
||||
sta_if.active(True)
|
||||
sta_if.connect(ssid, password)
|
||||
while not sta_if.isconnected():
|
||||
pass
|
||||
print("network config:", sta_if.ifconfig())
|
||||
|
||||
|
||||
# More info here: https://docs.micropython.org/en/latest/esp8266/tutorial/network_tcp.html
|
||||
def http_get(url):
|
||||
import socket
|
||||
|
||||
res = ""
|
||||
_, _, host, path = url.split("/", 3)
|
||||
addr = socket.getaddrinfo(host, 80)[0][-1]
|
||||
s = socket.socket()
|
||||
s.connect(addr)
|
||||
s.send(bytes("GET /%s HTTP/1.0\r\nHost: %s\r\n\r\n" % (path, host), "utf8"))
|
||||
while True:
|
||||
data = s.recv(100)
|
||||
if data:
|
||||
res += str(data, "utf8")
|
||||
else:
|
||||
break
|
||||
s.close()
|
||||
|
||||
return res
|
||||
|
||||
|
||||
# Calling functions defined above
|
||||
do_connect()
|
||||
response = http_get("http://micropython.org/ks/test.html")
|
||||
|
||||
# Initialise our Inkplate object
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
display.begin()
|
||||
|
||||
# Print response in lines
|
||||
cnt = 0
|
||||
for x in response.split("\n"):
|
||||
display.printText(
|
||||
10, 10 + cnt, x.upper()
|
||||
) # Default font has only upper case letters
|
||||
cnt += 10
|
||||
|
||||
# Display image from buffer
|
||||
display.display()
|
|
@ -0,0 +1,28 @@
|
|||
import os, time
|
||||
from soldered_inkplate6_PLUS import Inkplate
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_2BIT)
|
||||
display.begin()
|
||||
|
||||
# SD Card must be initialised with this function
|
||||
display.initSDCard()
|
||||
|
||||
# This prints all the files on card
|
||||
print(os.listdir("/sd"))
|
||||
|
||||
f = open("sd/text.txt", "r")
|
||||
|
||||
# Print file contents
|
||||
print(f.read())
|
||||
f.close()
|
||||
|
||||
time.sleep(5)
|
||||
|
||||
display.drawImageFile(0, 0, "sd/1.bmp")
|
||||
|
||||
# You can turn off the power to the SD card to save power
|
||||
display.SDCardSleep()
|
||||
# To turn it back on, use:
|
||||
# display.SDCardWake()
|
||||
|
||||
display.display()
|
|
@ -0,0 +1,73 @@
|
|||
import time
|
||||
from PCAL6416A import *
|
||||
|
||||
from soldered_inkplate6_PLUS import Inkplate
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
# This script demonstrates using all the available GPIO expander pins as output
|
||||
|
||||
if __name__ == "__main__":
|
||||
# Must be called before using, line in Arduino
|
||||
display.begin()
|
||||
|
||||
# pin = display.gpioExpanderPin(gpioExpander,pin,mode)
|
||||
# Supported gpio expanders on Soldered Inkplate 10: 1, 2
|
||||
# Supported modes: modeINPUT, modeINPUT_PULLUP, modeINPUT_PULLDOWN, modeOUTPUT
|
||||
# Supported pins on Soldered Inkplate 10 are listed below
|
||||
|
||||
expander1_P1_1 = display.gpioExpanderPin(1, 9, modeOUTPUT)
|
||||
expander1_P1_5 = display.gpioExpanderPin(1, 13, modeOUTPUT)
|
||||
expander1_P1_6 = display.gpioExpanderPin(1, 14, modeOUTPUT)
|
||||
expander1_P1_7 = display.gpioExpanderPin(1, 15, modeOUTPUT)
|
||||
|
||||
expander2_P0_0 = display.gpioExpanderPin(2, 0, modeOUTPUT)
|
||||
expander2_P0_1 = display.gpioExpanderPin(2, 1, modeOUTPUT)
|
||||
expander2_P0_2 = display.gpioExpanderPin(2, 2, modeOUTPUT)
|
||||
expander2_P0_3 = display.gpioExpanderPin(2, 3, modeOUTPUT)
|
||||
expander2_P0_4 = display.gpioExpanderPin(2, 4, modeOUTPUT)
|
||||
expander2_P0_5 = display.gpioExpanderPin(2, 5, modeOUTPUT)
|
||||
expander2_P0_6 = display.gpioExpanderPin(2, 6, modeOUTPUT)
|
||||
expander2_P0_7 = display.gpioExpanderPin(2, 7, modeOUTPUT)
|
||||
|
||||
expander2_P1_0 = display.gpioExpanderPin(2, 8, modeOUTPUT)
|
||||
expander2_P1_1 = display.gpioExpanderPin(2, 9, modeOUTPUT)
|
||||
expander2_P1_2 = display.gpioExpanderPin(2, 10, modeOUTPUT)
|
||||
expander2_P1_3 = display.gpioExpanderPin(2, 11, modeOUTPUT)
|
||||
expander2_P1_4 = display.gpioExpanderPin(2, 12, modeOUTPUT)
|
||||
expander2_P1_5 = display.gpioExpanderPin(2, 13, modeOUTPUT)
|
||||
expander2_P1_6 = display.gpioExpanderPin(2, 14, modeOUTPUT)
|
||||
expander2_P1_7 = display.gpioExpanderPin(2, 15, modeOUTPUT)
|
||||
|
||||
pins = (expander1_P1_1,
|
||||
expander1_P1_2,
|
||||
expander1_P1_3,
|
||||
expander1_P1_5,
|
||||
expander1_P1_6,
|
||||
expander1_P1_7,
|
||||
expander2_P0_0,
|
||||
expander2_P0_1,
|
||||
expander2_P0_2,
|
||||
expander2_P0_3,
|
||||
expander2_P0_4,
|
||||
expander2_P0_5,
|
||||
expander2_P0_6,
|
||||
expander2_P0_7,
|
||||
expander2_P1_0,
|
||||
expander2_P1_1,
|
||||
expander2_P1_2,
|
||||
expander2_P1_3,
|
||||
expander2_P1_4,
|
||||
expander2_P1_5,
|
||||
expander2_P1_6,
|
||||
expander2_P1_7,
|
||||
)
|
||||
|
||||
# This example writes a 0.2s pulse on the pins consecutively to test the output
|
||||
|
||||
while (1):
|
||||
for pin in pins:
|
||||
pin.digitalWrite(1)
|
||||
time.sleep(0.01)
|
||||
pin.digitalWrite(0)
|
||||
time.sleep(0.01)
|
|
@ -0,0 +1,22 @@
|
|||
from soldered_inkplate6_PLUS import Inkplate
|
||||
from image import *
|
||||
import time
|
||||
|
||||
display = Inkplate(Inkplate.INKPLATE_1BIT)
|
||||
|
||||
|
||||
#main function used by micropython
|
||||
if __name__ == "__main__":
|
||||
display.begin()
|
||||
display.tsInit(1)
|
||||
display.drawRect(450, 350, 100, 100, display.BLACK)
|
||||
display.display()
|
||||
|
||||
|
||||
counter = 0
|
||||
|
||||
while True:
|
||||
#touch the square
|
||||
if(display.touchInArea(450, 350, 100, 100)):
|
||||
counter += 1
|
||||
print(counter)
|
|
@ -0,0 +1,199 @@
|
|||
from machine import Pin as mPin
|
||||
from micropython import const
|
||||
|
||||
PCAL6416A_INPORT0 = const(0x00)
|
||||
PCAL6416A_INPORT1 = const(0x01)
|
||||
PCAL6416A_OUTPORT0 = const(0x02)
|
||||
PCAL6416A_OUTPORT1 = const(0x03)
|
||||
PCAL6416A_POLINVPORT0 = const(0x04)
|
||||
PCAL6416A_POLINVPORT1 = const(0x05)
|
||||
PCAL6416A_CFGPORT0 = const(0x06)
|
||||
PCAL6416A_CFGPORT1 = const(0x07)
|
||||
PCAL6416A_OUTDRVST_REG00 = const(0x40)
|
||||
PCAL6416A_OUTDRVST_REG01 = const(0x41)
|
||||
PCAL6416A_OUTDRVST_REG10 = const(0x42)
|
||||
PCAL6416A_OUTDRVST_REG11 = const(0x43)
|
||||
PCAL6416A_INLAT_REG0 = const(0x44)
|
||||
PCAL6416A_INLAT_REG1 = const(0x45)
|
||||
PCAL6416A_PUPDEN_REG0 = const(0x46)
|
||||
PCAL6416A_PUPDEN_REG1 = const(0x47)
|
||||
PCAL6416A_PUPDSEL_REG0 = const(0x48)
|
||||
PCAL6416A_PUPDSEL_REG1 = const(0x49)
|
||||
PCAL6416A_INTMSK_REG0 = const(0x4A)
|
||||
PCAL6416A_INTMSK_REG1 = const(0x4B)
|
||||
PCAL6416A_INTSTAT_REG0 = const(0x4C)
|
||||
PCAL6416A_INTSTAT_REG1 = const(0x4D)
|
||||
PCAL6416A_OUTPORT_CONF = const(0x4F)
|
||||
|
||||
PCAL6416A_INPORT0_ARRAY = const(0)
|
||||
PCAL6416A_INPORT1_ARRAY = const(1)
|
||||
PCAL6416A_OUTPORT0_ARRAY = const(2)
|
||||
PCAL6416A_OUTPORT1_ARRAY = const(3)
|
||||
PCAL6416A_POLINVPORT0_ARRAY = const(4)
|
||||
PCAL6416A_POLINVPORT1_ARRAY = const(5)
|
||||
PCAL6416A_CFGPORT0_ARRAY = const(6)
|
||||
PCAL6416A_CFGPORT1_ARRAY = const(7)
|
||||
PCAL6416A_OUTDRVST_REG00_ARRAY = const(8)
|
||||
PCAL6416A_OUTDRVST_REG01_ARRAY = const(9)
|
||||
PCAL6416A_OUTDRVST_REG10_ARRAY = const(10)
|
||||
PCAL6416A_OUTDRVST_REG11_ARRAY = const(11)
|
||||
PCAL6416A_INLAT_REG0_ARRAY = const(12)
|
||||
PCAL6416A_INLAT_REG1_ARRAY = const(13)
|
||||
PCAL6416A_PUPDEN_REG0_ARRAY = const(14)
|
||||
PCAL6416A_PUPDEN_REG1_ARRAY = const(15)
|
||||
PCAL6416A_PUPDSEL_REG0_ARRAY = const(16)
|
||||
PCAL6416A_PUPDSEL_REG1_ARRAY = const(17)
|
||||
PCAL6416A_INTMSK_REG0_ARRAY = const(18)
|
||||
PCAL6416A_INTMSK_REG1_ARRAY = const(19)
|
||||
PCAL6416A_INTSTAT_REG0_ARRAY = const(20)
|
||||
PCAL6416A_INTSTAT_REG1_ARRAY = const(21)
|
||||
PCAL6416A_OUTPORT_CONF_ARRAY = const(22)
|
||||
|
||||
IO_PIN_A0 = const(0)
|
||||
IO_PIN_A1 = const(1)
|
||||
IO_PIN_A2 = const(2)
|
||||
IO_PIN_A3 = const(3)
|
||||
IO_PIN_A4 = const(4)
|
||||
IO_PIN_A5 = const(5)
|
||||
IO_PIN_A6 = const(6)
|
||||
IO_PIN_A7 = const(7)
|
||||
IO_PIN_B0 = const(8)
|
||||
IO_PIN_B1 = const(9)
|
||||
IO_PIN_B2 = const(10)
|
||||
IO_PIN_B3 = const(11)
|
||||
IO_PIN_B4 = const(12)
|
||||
IO_PIN_B5 = const(13)
|
||||
IO_PIN_B6 = const(14)
|
||||
IO_PIN_B7 = const(15)
|
||||
|
||||
modeINPUT = const(0)
|
||||
modeOUTPUT = const(1)
|
||||
modeINPUT_PULLUP = const(2)
|
||||
modeINPUT_PULLDOWN = const(3)
|
||||
|
||||
|
||||
# PCAL6416A is a minimal driver for an 16-bit I2C I/O expander
|
||||
class PCAL6416A:
|
||||
def __init__(self, i2c, addr=0x20):
|
||||
self.i2c = i2c
|
||||
self.addr = addr
|
||||
self.ioRegsInt = bytearray(23)
|
||||
|
||||
self.ioRegsInt[0] = self.read(PCAL6416A_INPORT0)
|
||||
self.ioRegsInt[1] = self.read(PCAL6416A_INPORT1)
|
||||
self.ioRegsInt[2] = self.read(PCAL6416A_OUTPORT0)
|
||||
self.ioRegsInt[3] = self.read(PCAL6416A_OUTPORT1)
|
||||
self.ioRegsInt[4] = self.read(PCAL6416A_POLINVPORT0)
|
||||
self.ioRegsInt[5] = self.read(PCAL6416A_POLINVPORT1)
|
||||
self.ioRegsInt[6] = self.read(PCAL6416A_CFGPORT0)
|
||||
self.ioRegsInt[7] = self.read(PCAL6416A_CFGPORT1)
|
||||
self.ioRegsInt[8] = self.read(PCAL6416A_OUTDRVST_REG00)
|
||||
self.ioRegsInt[9] = self.read(PCAL6416A_OUTDRVST_REG01)
|
||||
self.ioRegsInt[10] = self.read(PCAL6416A_OUTDRVST_REG10)
|
||||
self.ioRegsInt[11] = self.read(PCAL6416A_OUTDRVST_REG11)
|
||||
self.ioRegsInt[12] = self.read(PCAL6416A_INLAT_REG0)
|
||||
self.ioRegsInt[13] = self.read(PCAL6416A_INLAT_REG1)
|
||||
self.ioRegsInt[14] = self.read(PCAL6416A_PUPDEN_REG0)
|
||||
self.ioRegsInt[15] = self.read(PCAL6416A_PUPDEN_REG1)
|
||||
self.ioRegsInt[16] = self.read(PCAL6416A_PUPDSEL_REG0)
|
||||
self.ioRegsInt[17] = self.read(PCAL6416A_PUPDSEL_REG1)
|
||||
self.ioRegsInt[18] = self.read(PCAL6416A_INTMSK_REG0)
|
||||
self.ioRegsInt[19] = self.read(PCAL6416A_INTMSK_REG1)
|
||||
self.ioRegsInt[20] = self.read(PCAL6416A_INTSTAT_REG0)
|
||||
self.ioRegsInt[21] = self.read(PCAL6416A_INTSTAT_REG1)
|
||||
self.ioRegsInt[22] = self.read(PCAL6416A_OUTPORT_CONF)
|
||||
|
||||
# read an 8-bit register, internal method
|
||||
def read(self, reg):
|
||||
return self.i2c.readfrom_mem(self.addr, reg, 1)[0]
|
||||
|
||||
# write an 8-bit register, internal method
|
||||
def write(self, reg, v):
|
||||
self.i2c.writeto_mem(self.addr, reg, bytes((v,)))
|
||||
|
||||
# write two 8-bit registers, internal method
|
||||
def write2(self, reg, v1, v2):
|
||||
self.i2c.writeto_mem(self.addr, reg, bytes((v1, v2)))
|
||||
|
||||
# writebuf writes multiple bytes to the same register
|
||||
def writebuf(self, reg, v):
|
||||
self.i2c.writeto_mem(self.addr, reg, v)
|
||||
|
||||
def pinMode(self, pin, mode):
|
||||
|
||||
if (pin > 15):
|
||||
return
|
||||
|
||||
port = pin // 8
|
||||
pin = pin % 8
|
||||
|
||||
if (mode == modeINPUT):
|
||||
self.ioRegsInt[PCAL6416A_CFGPORT0_ARRAY + port] |= (1 << pin)
|
||||
self.write(PCAL6416A_CFGPORT0 + port,
|
||||
self.ioRegsInt[PCAL6416A_CFGPORT0_ARRAY + port])
|
||||
elif (mode == modeOUTPUT):
|
||||
self.ioRegsInt[PCAL6416A_CFGPORT0_ARRAY + port] &= ~ (1 << pin)
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port] &= ~(1 << pin)
|
||||
self.write(PCAL6416A_OUTPORT0 + port,
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port])
|
||||
self.write(PCAL6416A_CFGPORT0 + port,
|
||||
self.ioRegsInt[PCAL6416A_CFGPORT0_ARRAY + port])
|
||||
elif (mode == modeINPUT_PULLUP):
|
||||
self.ioRegsInt[PCAL6416A_CFGPORT0_ARRAY + port] |= (1 << pin)
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port] |= (1 << pin)
|
||||
self.ioRegsInt[PCAL6416A_PUPDSEL_REG0_ARRAY + port] |= (1 << pin)
|
||||
self.write(PCAL6416A_OUTPORT0 + port,
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port])
|
||||
self.write(PCAL6416A_CFGPORT0 + port,
|
||||
self.ioRegsInt[PCAL6416A_CFGPORT0_ARRAY + port])
|
||||
self.write(PCAL6416A_PUPDSEL_REG0 + port,
|
||||
self.ioRegsInt[PCAL6416A_PUPDSEL_REG0_ARRAY + port])
|
||||
elif (mode == modeINPUT_PULLDOWN):
|
||||
self.ioRegsInt[PCAL6416A_CFGPORT0_ARRAY + port] |= (1 << pin)
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port] |= (1 << pin)
|
||||
self.ioRegsInt[PCAL6416A_PUPDSEL_REG0_ARRAY + port] &= ~(1 << pin)
|
||||
self.write(PCAL6416A_OUTPORT0 + port,
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port])
|
||||
self.write(PCAL6416A_CFGPORT0 + port,
|
||||
self.ioRegsInt[PCAL6416A_CFGPORT0_ARRAY + port])
|
||||
self.write(PCAL6416A_PUPDSEL_REG0 + port,
|
||||
self.ioRegsInt[PCAL6416A_PUPDSEL_REG0_ARRAY + port])
|
||||
|
||||
def digitalWrite(self, pin, state):
|
||||
if (pin > 15):
|
||||
return
|
||||
|
||||
state &= 1
|
||||
port = pin // 8
|
||||
pin %= 8
|
||||
|
||||
if (state):
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port] |= (1 << pin)
|
||||
else:
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port] &= ~(1 << pin)
|
||||
|
||||
self.write(PCAL6416A_OUTPORT0 + port,
|
||||
self.ioRegsInt[PCAL6416A_OUTPORT0_ARRAY + port])
|
||||
|
||||
def digitalRead(self, pin):
|
||||
if (pin > 15):
|
||||
return
|
||||
|
||||
port = pin // 8
|
||||
pin %= 8
|
||||
|
||||
self.ioRegsInt[PCAL6416A_INPORT0_ARRAY +
|
||||
port] = self.read(PCAL6416A_INPORT0_ARRAY + port)
|
||||
return (self.ioRegsInt[PCAL6416A_INPORT0 + port] >> pin) & 1
|
||||
|
||||
class gpioPin:
|
||||
def __init__(self, PCAL6416A, pin, mode):
|
||||
self.PCAL6416A = PCAL6416A
|
||||
self.pin = pin
|
||||
self.PCAL6416A.pinMode(pin,mode)
|
||||
|
||||
def digitalWrite(self, value):
|
||||
self.PCAL6416A.digitalWrite(self.pin,value)
|
||||
|
||||
def digitalRead(self):
|
||||
return self.PCAL6416A.digitalRead(self.pin)
|
168
README.md
168
README.md
|
@ -1,105 +1,125 @@
|
|||
# Inkplate micropython module
|
||||
# Soldered Inkplate Micropython library
|
||||
|
||||
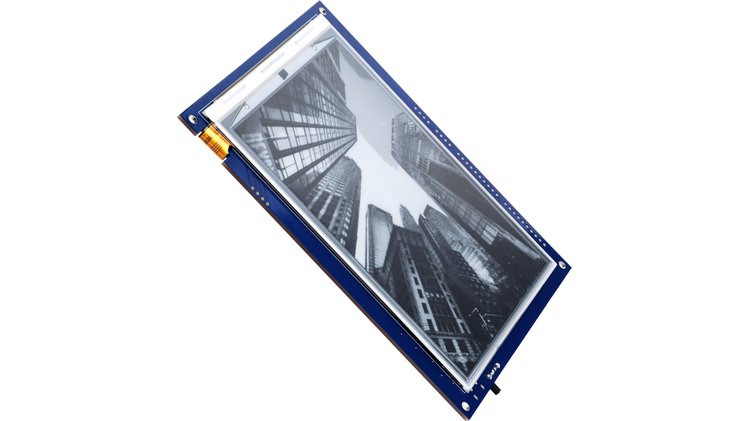
|
||||
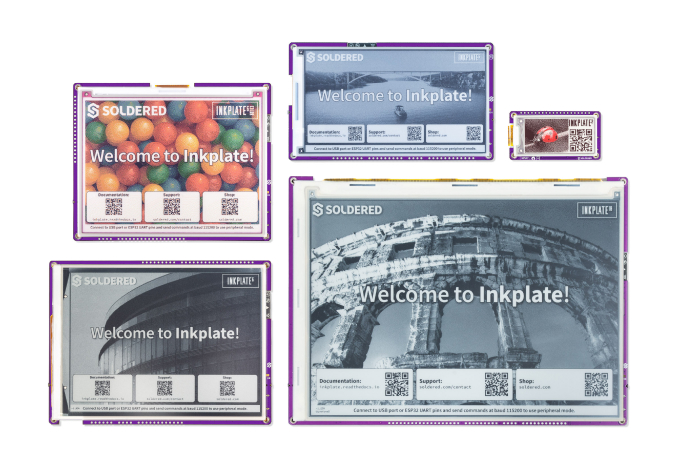
|
||||
|
||||
Micropython for all-in-one e-paper display Inkplate can be found in this repo. Inkplate is a powerful, Wi-Fi enabled ESP32 based e-paper display – recycled from a Kindle e-reader. Its main feature is simplicity. Just plug in a USB cable, open Arduino IDE, and change the contents of the screen with few lines of code. Learn more about Inkplate on [official website](https://inkplate.io/). Inkplate was crowdfunded on [Crowd Supply Inkplate 6](https://www.crowdsupply.com/e-radionica/inkplate-6) and [Crowd Supply Inkplate 10](https://www.crowdsupply.com/e-radionica/inkplate-10).
|
||||
The Micropython modules for the Inkplate product family can befound in this repository. Inkplate is a series of powerful, Wi-Fi and Bluetooth enabled, ESP32-based ePaper display products. Its main feature is simplicity. Just plug in a USB cable, load the MicroPython firmware and the required libraries and run your script on Inkplate itself. The Inkplate product family currently includes Inkplate 10, Inkplate 6 and Inkplate 6PLUS, Inkplate 6COLOR and Inkplate 2.
|
||||
Inkplate 6 was crowdfunded on [Crowd Supply](https://www.crowdsupply.com/e-radionica/inkplate-6), as well as [Inkplate 10](https://www.crowdsupply.com/e-radionica/inkplate-10), [Inkplate 6PLUS](https://www.crowdsupply.com/e-radionica/inkplate-6plus) and [Inkplate 6COLOR](https://www.crowdsupply.com/soldered/inkplate-6color). Inkplate 2 was funded on [Kickstarter](https://www.kickstarter.com/projects/solderedelectronics/inkplate-2-a-easy-to-use-arduino-compatible-e-paper).
|
||||
|
||||
Original effort done by [tve](https://github.com/tve/micropython-inkplate6).
|
||||
All available to purchase from [soldered.com](https://soldered.com/categories/inkplate/).
|
||||
|
||||
### Features
|
||||
Original effort to enable MicroPython support for Inkplate was done by [tve](https://github.com/tve/micropython-inkplate6). Thank you!
|
||||
|
||||
- Simple graphics class for monochrome use of the e-paper display
|
||||
- Simple graphics class for 2 bits per pixel greyscale use of the e-paper display
|
||||
- Support for partial updates (currently only on the monochrome display)
|
||||
- Access to touch sensors
|
||||
- Everything in pure python with screen updates virtually as fast as the Arduino C driver
|
||||
- Bitmap drawing, although really slow one
|
||||
### Setting up Inkplate with MicroPython
|
||||
|
||||
### Getting started with micropython on Inkplate
|
||||
In order to get started with running your code on Inkplate, follow these steps:
|
||||
1. Install esptool - the command line tool used to upload firmware to the ESP32. Get it from [here](https://github.com/espressif/esptool) (https://github.com/espressif/esptool). Also, install PySerial as it's a requirement. You can download PySerial [here](https://pypi.org/project/pyserial/) (https://pypi.org/project/pyserial). Place them in a both in a working directory.
|
||||
|
||||
- Flash MicroPython firmware for **ESP32 with SPIRAM** from https://micropython.org/download/esp32spiram/
|
||||
- Port name/number vary on different devices
|
||||
- Run
|
||||
```
|
||||
//Linux/Mac
|
||||
esptool.py --port /dev/cu.usbserial-1420 erase_flash
|
||||
2. Download or clone this repository by clicking Code -> Download as .zip. Extract to your desired working directory for your MicroPython files, make it a different one than the esptool directory.
|
||||
|
||||
//Windows
|
||||
esptool.py --port COM5 erase_flash
|
||||
```
|
||||
to erase esp32 flash and then
|
||||
```
|
||||
//Linux/Mac
|
||||
3. Copy the esp32spiram-20220117-v1.18.bin file to the esptool directory from the MicroPython directory. Open your terminal/command prompt in the esptool directory.
|
||||
|
||||
esptool.py --chip esp32 --port /dev/cu.usbserial-1420 write_flash -z 0x1000 esp32spiram-20220117-v1.18.bin
|
||||
4. We need to flash the MicroPython firmware to Inkplate. It is reccomended to flash the one supplied in this repository that you have copied in the previous step, version 1.18. To do this, connect Inkplate via USB-C and first erase the flash memory by running this command:
|
||||
```
|
||||
//Linux/Mac
|
||||
python3 esptool.py --port /dev/cu.usbserial-1420 erase_flash
|
||||
|
||||
//Windows
|
||||
esptool.py --chip esp32 --port COM5 write_flash -z 0x1000 esp32spiram-20220117-v1.18.bin
|
||||
```
|
||||
to flash supplied firmware. Use esp flash from this repository since it's tested with Inkplate.
|
||||
|
||||
If you don't have esptool.py installed, install it from here: https://github.com/espressif/esptool, at minimum use version 1.15.
|
||||
//Windows
|
||||
python esptool.py --port COM5 erase_flash
|
||||
```
|
||||
**NOTE:** You should change the serial port listed here to the one which corresponds to your connected Inkplate device.
|
||||
|
||||
- Copy library files to your board, use inkplate6.py or inkplate10.py for respective versions, something like this:
|
||||
```
|
||||
//Linux/Mac
|
||||
python3 pyboard.py --device /dev/ttyUSB0 -f cp mcp23017.py inkplate6.py image.py shapes.py gfx.py gfx_standard_font_01.py :
|
||||
Now it's possible to flash MicroPython firmware. Do so by running this command:
|
||||
```
|
||||
//Linux/Mac
|
||||
python3 esptool.py --chip esp32 --port /dev/cu.usbserial-1420 write_flash -z 0x1000 esp32spiram-20220117-v1.18.bin
|
||||
// If you're having problems on Mac, use a slower baud rate with the flag "-b 112500"
|
||||
|
||||
//Windows
|
||||
//This one might need to be started twice
|
||||
python pyboard.py --device COM5 -f cp inkplate6.py gfx.py gfx_standard_font_01.py mcp23017.py image.py shapes.py :
|
||||
```
|
||||
(You can find `pyboard.py` in the MicroPython tools directory or just download it from
|
||||
GitHub: https://raw.githubusercontent.com/micropython/micropython/master/tools/pyboard.py)
|
||||
//Windows
|
||||
python esptool.py --chip esp32 --port COM5 write_flash -z 0x1000 esp32spiram-20220117-v1.18.bin
|
||||
```
|
||||
|
||||
- Run `example.py`:
|
||||
```
|
||||
//Linux/Mac
|
||||
python3 pyboard.py --device /dev/ttyUSB0 "Examples/Inkplate6/basicBW.py"
|
||||
**You only have to do steps 1-4 once when writing MicroPython firmware on your Inkplate!** If you have already done this, proceed from step 5 onwards.
|
||||
|
||||
//Windows
|
||||
python pyboard.py --device COM5 "Examples/Inkplate6/basicBW.py"
|
||||
```
|
||||
- You can run our others examples, showing how to use rest of the functionalities.
|
||||
5. Open a terminal in your MicroPython folder. Now, it's required to copy all the library files and drivers for your Inkplate board, so your MicroPython script can run. Do so with the following command:
|
||||
|
||||
### Examples
|
||||
```
|
||||
//Linux/Mac
|
||||
python3 pyboard.py --device /dev/ttyUSB0 -f cp mcp23017.py inkplate6.py image.py shapes.py gfx.py gfx_standard_font_01.py :
|
||||
|
||||
The repo contains many examples which can demonstrate the Inkplate capabilities.
|
||||
- basicBW.py -> demonstrates basic drawing capabilities, as well as drawing some images.
|
||||
- basicGrayscale.py -> demonstrates basic drawing capabilities, as well as drawing some images.
|
||||
- exampleNetwork.py -> demonstrates connection to WiFi network while drawing the HTTP request response on the screen.
|
||||
- exampleSd.py -> demonstrates reading files and images from SD card.
|
||||
- batteryAndTemperatureRead.py -> demonstrates how to read temperature and voltage from internal sensors.
|
||||
- touchpads.py -> demonstrates how to use built in touchpads.
|
||||
//Windows
|
||||
//This one might need to be started twice
|
||||
python pyboard.py --device COM5 -f cp inkplate6.py gfx.py gfx_standard_font_01.py mcp23017.py image.py shapes.py :
|
||||
```
|
||||
|
||||
**NOTE:** here you need to again change the serial port to the one you're using and the main driver of the board to the one made specifically for your Inkplate board. Here it's inkplate6.py for Inkplate 6. If you have a newer version of Inkplate 6 (Soldered Inkplate 6) then copy soldered_inkplate6.py instead. inkplate2.py for Inkplate 2, and so on.
|
||||
|
||||
In this command you also need to include all the files your Python script uses (external images, files with extenral functions you're including and so on) so it can run on your board!
|
||||
|
||||
7. Finally, it's time to run the MicroPython script which will actually run on the device. To demonstrate, we will run the basicBW.py example for Inkplate 6. To run the script, execute the following command:
|
||||
|
||||
```
|
||||
//Linux/Mac
|
||||
python3 pyboard.py --device /dev/ttyUSB0 "Examples/Inkplate6/basicBW.py"
|
||||
|
||||
//Windows
|
||||
python pyboard.py --device COM5 "Examples/Inkplate6/basicBW.py"
|
||||
```
|
||||
|
||||
You can try other examples which will show you all the features of the device.
|
||||
|
||||
|
||||
### Code examples
|
||||
|
||||
There are several examples which will indicate all the functions you can use in your own script:
|
||||
* The basic examples show you drawing shapes, lines and text on the screen in different colors, also a bitmap image in a single color (color image drawing with dithering will be supported soon!)
|
||||
* The network examples show you how to use the network features like doing a GET request and downloading a file
|
||||
* The batteryAndTemperatureRead examples show you how to read the internal battery status and the temperature from the internal sensor
|
||||
* The exampleSD example shows you how to read image files and text from the SD card
|
||||
* The gpio_expander example shows how to use the GPIO expander on new Inkplate models
|
||||
* The touchpad examples show you how to use the touchpad on older Inkplates
|
||||
|
||||
### Documentation
|
||||
|
||||
Find Inkplate documentation [here](https://inkplate.readthedocs.io/).
|
||||
|
||||
### Battery power
|
||||
|
||||
Inkplate has two options for powering it. First one is obvious - USB port at side of the board. Just plug any micro USB cable and you are good to go. Second option is battery. Supported batteries are standard Li-Ion/Li-Poly batteries with 3.7V nominal voltage. Connector for the battery is standard 2.00mm pitch JST connector. The onboard charger will charge the battery with 500mA when USB is plugged at the same time. You can use battery of any size or capacity if you don't have a enclosure. If you are using our enclosure, battery size shouldn't exceed 90mm x 40mm (3.5 x 1.57 inch) and 5mm (0.19 inch) in height. [This battery](https://e-radionica.com/en/li-ion-baterija-1200mah.html) is good fit for the Inkplate.
|
||||
Inkplate boards has two options for powering it. First one is obvious - USB port at side of the board. Just plug any micro USB cable and you are good to go. Second option is battery. Supported batteries are standard Li-Ion/Li-Poly batteries with 3.7V nominal voltage. Connector for the battery is standard 2.00mm pitch JST connector (except on Inkplate 2, it uses SMD solder pads for battery terminals). The onboard charger will charge the battery with 500mA when USB is plugged at the same time. You can use battery of any size or capacity if you don't have a enclosure. If you are using our enclosure, battery size shouldn't exceed 90mm x 40mm (3.5 x 1.57 inch) and 5mm (0.19 inch) in height (excluding Inkplate 2, it uses [this battery](https://soldered.com/product/li-ion-baterija-600mah-3-7v/). [This battery](https://soldered.com/product/li-ion-battery-1200mah-3-7v/) is good fit for the Inkplate. Also, Inkplate's hardware is specially optimized for low power consumption in deep sleep mode, making it extremely suitable for battery applications.
|
||||
|
||||
### Arduino?
|
||||
#### ⚠️ WARNING
|
||||
Please check the polarity on the battery JST connector! Some batteries that can be purchased from the web have reversed polarity that can damage Inkplate board! You are safe if you are using the pouch battery from [soldered.com](https://soldered.com/categories/power-sources-batteries/batteries/lithium-batteries/) or Inkplate with the built-in battery .
|
||||
|
||||
Looking for Arduino library? Look [here](https://github.com/e-radionicacom/Inkplate-6-Arduino-library)!
|
||||
#### ℹ NOTE
|
||||
CR2032 battery is only for RTC backup. Inkplate cannot be powered with it.
|
||||
|
||||
### License
|
||||
|
||||
This repo is licensed with the MIT License. For more info, see LICENSE.
|
||||
|
||||
### Open-source
|
||||
|
||||
All of Inkplate-related development is open-sourced:
|
||||
- [Arduino library](https://github.com/e-radionicacom/Inkplate-6-Arduino-library)
|
||||
- [Inkplate 6 hardware](https://github.com/e-radionicacom/Inkplate-6-hardware)
|
||||
- [micropython Inkplate](https://github.com/e-radionicacom/Inkplate-6-micropython)
|
||||
- [OSHWA certificate](https://certification.oshwa.org/hr000003.html)
|
||||
|
||||
- [Arduino library](https://github.com/SolderedElectronics/Inkplate-Arduino-library)
|
||||
- Hardware design:
|
||||
- Soldered Inkplate 2 (comming soon!)
|
||||
- Soldered Inkplate 6 (comming soon!)
|
||||
- Soldered Inkplate 6PLUS (comming soon!)
|
||||
- [Soldered Inkplate 10](https://github.com/SolderedElectronics/Soldered-Inkplate-10-hardware-design)
|
||||
- Soldered Inkplate 6COLOR (comming soon!)
|
||||
- [e-radionica.com Inkplate 6](https://github.com/SolderedElectronics/Inkplate-6-hardware)
|
||||
- [e-radionica.com Inkplate 10](https://github.com/SolderedElectronics/Inkplate-10-hardware)
|
||||
- [e-radionica.com Inkplate 6PLUS](https://github.com/SolderedElectronics/Inkplate-6PLUS-Hardware)
|
||||
- e-radionica.com Inkplate 6COLOR (comming soon!)
|
||||
- [micropython Inkplate](https://github.com/SolderedElectronics/Inkplate-micropython)
|
||||
- [OSHWA cerfiticates](https://certification.oshwa.org/list.html?q=inkplate)
|
||||
|
||||
### Where to buy & other
|
||||
|
||||
Inkplate is available for purchase via:
|
||||
Inkplate boards are available for purchase via:
|
||||
|
||||
- [e-radionica.com](https://e-radionica.com/en/inkplate.html)
|
||||
- [Crowd Supply Inkplate 6](https://www.crowdsupply.com/e-radionica/inkplate-6)
|
||||
- [Crowd Supply Inkplate 10](https://www.crowdsupply.com/e-radionica/inkplate-10)
|
||||
- [soldered.com](https://soldered.com/categories/inkplate/)
|
||||
- [Crowd Supply](https://www.crowdsupply.com/soldered)
|
||||
- [Mouser](https://hr.mouser.com/Search/Refine?Keyword=inkplate)
|
||||
- [Sparkfun](https://www.sparkfun.com/search/results?term=inkplate)
|
||||
- [Pimoroni](https://shop.pimoroni.com/products/inkplate-6)
|
||||
|
||||
Inkplate is open-source. If you are looking for hardware design of the board, check the [Hardware repo for Inkplate 6](https://github.com/e-radionicacom/Inkplate-6-hardware) and [Hardware repo for Inkplate 10](https://github.com/e-radionicacom/Inkplate-10-hardware). You will find 3D printable [enclosure](https://github.com/e-radionicacom/Inkplate-6-hardware/tree/master/3D%20printable%20case) there, as well as [detailed dimensions](https://github.com/e-radionicacom/Inkplate-6-hardware/tree/master/Technical%20drawings). In this repo you will find code for driving the ED060SC7 e-paper display used by Inkplate.
|
||||
|
||||
For all questions and issues, please use our [forum](https://forum.e-radionica.com/en/) to ask a question.
|
||||
For sales & collaboration, please reach us via [e-mail](mailto:kontakt@e-radionica.com).
|
||||
For all questions and issues please reach us via [e-mail](mailto:hello@soldered.com) or our [contact form](https://soldered.com/contact/).
|
|
@ -0,0 +1,372 @@
|
|||
import time
|
||||
import os
|
||||
from machine import ADC, I2C, SPI, Pin
|
||||
from micropython import const
|
||||
from shapes import Shapes
|
||||
from machine import Pin as mPin
|
||||
from gfx import GFX
|
||||
from gfx_standard_font_01 import text_dict as std_font
|
||||
|
||||
# Connections between ESP32 and color Epaper
|
||||
EPAPER_RST_PIN = const(19)
|
||||
EPAPER_DC_PIN = const(33)
|
||||
EPAPER_CS_PIN = const(27)
|
||||
EPAPER_BUSY_PIN = const(32)
|
||||
EPAPER_CLK = const(18)
|
||||
EPAPER_DIN = const(23)
|
||||
|
||||
pixelMaskLUT = [0x1, 0x2, 0x4, 0x8, 0x10, 0x20, 0x40, 0x80]
|
||||
|
||||
# ePaper resolution
|
||||
# For Inkplate2 height and width are swapped in relation to the default rotation
|
||||
E_INK_HEIGHT = 212
|
||||
E_INK_WIDTH = 104
|
||||
|
||||
E_INK_NUM_PIXELS = E_INK_HEIGHT * E_INK_WIDTH
|
||||
E_INK_BUFFER_SIZE = E_INK_NUM_PIXELS // 8
|
||||
|
||||
busy_timeout_ms = 30000
|
||||
|
||||
|
||||
class Inkplate:
|
||||
|
||||
# Colors
|
||||
WHITE = 0b00000000
|
||||
BLACK = 0b00000001
|
||||
RED = 0b00000010
|
||||
|
||||
_width = E_INK_WIDTH
|
||||
_height = E_INK_HEIGHT
|
||||
|
||||
rotation = 0
|
||||
textSize = 1
|
||||
|
||||
_panelState = False
|
||||
|
||||
_framebuf_BW = bytearray([0xFF] * E_INK_BUFFER_SIZE)
|
||||
_framebuf_RED = bytearray([0xFF] * E_INK_BUFFER_SIZE)
|
||||
|
||||
@classmethod
|
||||
def begin(self):
|
||||
self.wire = I2C(0, scl=Pin(22), sda=Pin(21))
|
||||
self.spi = SPI(2)
|
||||
self._framebuf_BW = bytearray(([0xFF] * E_INK_BUFFER_SIZE))
|
||||
self._framebuf_RED = bytearray(([0xFF] * E_INK_BUFFER_SIZE))
|
||||
|
||||
self.GFX = GFX(
|
||||
E_INK_HEIGHT,
|
||||
E_INK_WIDTH,
|
||||
self.writePixel,
|
||||
self.writeFastHLine,
|
||||
self.writeFastVLine,
|
||||
self.writeFillRect,
|
||||
None,
|
||||
None,
|
||||
)
|
||||
|
||||
# Wake the panel and init it
|
||||
if not (self.setPanelDeepSleepState(False)):
|
||||
return False
|
||||
|
||||
# Put it back to sleep
|
||||
self.setPanelDeepSleepState(True)
|
||||
|
||||
# 3 is the default rotation for Inkplate 2
|
||||
self.setRotation(3)
|
||||
|
||||
return True
|
||||
|
||||
@classmethod
|
||||
def getPanelDeepSleepState(self):
|
||||
return self._panelState
|
||||
|
||||
@classmethod
|
||||
def setPanelDeepSleepState(self, state):
|
||||
|
||||
# False wakes the panel up
|
||||
# True puts it to sleep
|
||||
if not state:
|
||||
self.spi.init(baudrate=20000000, firstbit=SPI.MSB,
|
||||
polarity=0, phase=0)
|
||||
self.EPAPER_BUSY_PIN = Pin(EPAPER_BUSY_PIN, Pin.IN)
|
||||
self.EPAPER_RST_PIN = Pin(EPAPER_RST_PIN, Pin.OUT)
|
||||
self.EPAPER_DC_PIN = Pin(EPAPER_DC_PIN, Pin.OUT)
|
||||
self.EPAPER_CS_PIN = Pin(EPAPER_CS_PIN, Pin.OUT)
|
||||
time.sleep_ms(10)
|
||||
self.resetPanel()
|
||||
|
||||
# Reinit the panel
|
||||
self.sendCommand(b"\x04")
|
||||
_timeout = time.ticks_ms()
|
||||
while not self.EPAPER_BUSY_PIN.value() and (time.ticks_ms() - _timeout) < busy_timeout_ms:
|
||||
pass
|
||||
|
||||
self.sendCommand(b"\x00")
|
||||
self.sendData(b"\x0f")
|
||||
self.sendData(b"\x89")
|
||||
self.sendCommand(b"\x61")
|
||||
self.sendData(b"\x68")
|
||||
self.sendData(b"\x00")
|
||||
self.sendData(b"\xD4")
|
||||
self.sendCommand(b"\x50")
|
||||
self.sendData(b"\x77")
|
||||
|
||||
self._panelState = True
|
||||
|
||||
return True
|
||||
|
||||
else:
|
||||
|
||||
# Put the panel to sleep
|
||||
self.sendCommand(b"\x50")
|
||||
self.sendData(b"\xf7")
|
||||
self.sendCommand(b"\x02")
|
||||
# Wait for ePaper
|
||||
_timeout = time.ticks_ms()
|
||||
while not self.EPAPER_BUSY_PIN.value() and (time.ticks_ms() - _timeout) < busy_timeout_ms:
|
||||
pass
|
||||
self.sendCommand(b"\07")
|
||||
self.sendData(b"\xA5")
|
||||
|
||||
time.sleep_ms(1)
|
||||
# Turn off SPI
|
||||
self.spi.deinit()
|
||||
self.EPAPER_BUSY_PIN = Pin(EPAPER_BUSY_PIN, Pin.IN)
|
||||
self.EPAPER_RST_PIN = Pin(EPAPER_RST_PIN, Pin.IN)
|
||||
self.EPAPER_DC_PIN = Pin(EPAPER_DC_PIN, Pin.IN)
|
||||
self.EPAPER_CS_PIN = Pin(EPAPER_CS_PIN, Pin.IN)
|
||||
|
||||
self._panelState = False
|
||||
|
||||
return False
|
||||
|
||||
@classmethod
|
||||
def resetPanel(self):
|
||||
self.EPAPER_RST_PIN.value(0)
|
||||
time.sleep_ms(10)
|
||||
self.EPAPER_RST_PIN.value(1)
|
||||
time.sleep_ms(10)
|
||||
|
||||
@classmethod
|
||||
def sendCommand(self, command):
|
||||
self.EPAPER_DC_PIN.value(0)
|
||||
self.EPAPER_CS_PIN.value(0)
|
||||
self.spi.write(command)
|
||||
|
||||
self.EPAPER_CS_PIN.value(1)
|
||||
|
||||
@classmethod
|
||||
def sendData(self, data):
|
||||
self.EPAPER_CS_PIN.value(0)
|
||||
self.EPAPER_DC_PIN.value(1)
|
||||
self.spi.write(data)
|
||||
|
||||
self.EPAPER_CS_PIN.value(1)
|
||||
time.sleep_ms(1)
|
||||
|
||||
@classmethod
|
||||
def clearDisplay(self):
|
||||
self._framebuf_BW = bytearray(([0xFF] * E_INK_BUFFER_SIZE))
|
||||
self._framebuf_RED = bytearray(([0xFF] * E_INK_BUFFER_SIZE))
|
||||
|
||||
@classmethod
|
||||
def display(self):
|
||||
|
||||
# Wake the display
|
||||
self.setPanelDeepSleepState(False)
|
||||
|
||||
# Write b/w pixels
|
||||
self.sendCommand(b"\x10")
|
||||
self.sendData(self._framebuf_BW)
|
||||
|
||||
# Write red pixels
|
||||
self.sendCommand(b"\x13")
|
||||
self.sendData(self._framebuf_RED)
|
||||
|
||||
# Stop transfer
|
||||
self.sendCommand(b"\x11")
|
||||
self.sendData(b"\x00")
|
||||
|
||||
# Refresh
|
||||
self.sendCommand(b"\x12")
|
||||
time.sleep_ms(5)
|
||||
|
||||
_timeout = time.ticks_ms()
|
||||
while not self.EPAPER_BUSY_PIN.value() and (time.ticks_ms() - _timeout) < busy_timeout_ms:
|
||||
pass
|
||||
|
||||
# Put the display back to sleep
|
||||
self.setPanelDeepSleepState(True)
|
||||
|
||||
@classmethod
|
||||
def width(self):
|
||||
return self._width
|
||||
|
||||
@classmethod
|
||||
def height(self):
|
||||
return self._height
|
||||
|
||||
# Arduino compatibility functions
|
||||
@classmethod
|
||||
def setRotation(self, x):
|
||||
self.rotation = x % 4
|
||||
if self.rotation == 0 or self.rotation == 2:
|
||||
self._width = E_INK_WIDTH
|
||||
self._height = E_INK_HEIGHT
|
||||
elif self.rotation == 1 or self.rotation == 3:
|
||||
self._width = E_INK_HEIGHT
|
||||
self._height = E_INK_WIDTH
|
||||
|
||||
@classmethod
|
||||
def getRotation(self):
|
||||
return self.rotation
|
||||
|
||||
@classmethod
|
||||
def drawPixel(self, x, y, c):
|
||||
self.startWrite()
|
||||
self.writePixel(x, y, c)
|
||||
self.endWrite()
|
||||
|
||||
@classmethod
|
||||
def startWrite(self):
|
||||
pass
|
||||
|
||||
@classmethod
|
||||
def writePixel(self, x, y, c):
|
||||
if x > self.width() - 1 or y > self.height() - 1 or x < 0 or y < 0:
|
||||
return
|
||||
if (c > 2):
|
||||
return
|
||||
|
||||
if self.rotation == 3:
|
||||
x, y = y, x
|
||||
y = self.width() - y - 1
|
||||
elif self.rotation == 0:
|
||||
x = self.width() - x - 1
|
||||
y = self.height() - y - 1
|
||||
elif self.rotation == 1:
|
||||
x, y = y, x
|
||||
x = self.height() - x - 1
|
||||
elif self.rotation == 2:
|
||||
pass
|
||||
|
||||
_x = x // 8
|
||||
_x_sub = x % 8
|
||||
_position = E_INK_WIDTH // 8 * y + _x
|
||||
|
||||
# Clear both black and red frame buffer
|
||||
self._framebuf_BW[_position] |= (pixelMaskLUT[7 - _x_sub])
|
||||
self._framebuf_RED[_position] |= (pixelMaskLUT[7 - _x_sub])
|
||||
|
||||
# Write the pixel to the according buffer
|
||||
if (c < 2):
|
||||
self._framebuf_BW[_position] &= ~(c << (7 - _x_sub))
|
||||
else:
|
||||
self._framebuf_RED[_position] &= ~(pixelMaskLUT[7 - _x_sub])
|
||||
|
||||
@classmethod
|
||||
def writeFillRect(self, x, y, w, h, c):
|
||||
for j in range(w):
|
||||
for i in range(h):
|
||||
self.writePixel(x + j, y + i, c)
|
||||
|
||||
@classmethod
|
||||
def writeFastVLine(self, x, y, h, c):
|
||||
for i in range(h):
|
||||
self.writePixel(x, y + i, c)
|
||||
|
||||
@classmethod
|
||||
def writeFastHLine(self, x, y, w, c):
|
||||
for i in range(w):
|
||||
self.writePixel(x + i, y, c)
|
||||
|
||||
@classmethod
|
||||
def writeLine(self, x0, y0, x1, y1, c):
|
||||
self.GFX.line(x0, y0, x1, y1, c)
|
||||
|
||||
@classmethod
|
||||
def endWrite(self):
|
||||
pass
|
||||
|
||||
@classmethod
|
||||
def drawFastVLine(self, x, y, h, c):
|
||||
self.startWrite()
|
||||
self.writeFastVLine(x, y, h, c)
|
||||
self.endWrite()
|
||||
|
||||
@classmethod
|
||||
def drawFastHLine(self, x, y, w, c):
|
||||
self.startWrite()
|
||||
self.writeFastHLine(x, y, w, c)
|
||||
self.endWrite()
|
||||
|
||||
@classmethod
|
||||
def fillRect(self, x, y, w, h, c):
|
||||
self.startWrite()
|
||||
self.writeFillRect(x, y, w, h, c)
|
||||
self.endWrite()
|
||||
|
||||
@classmethod
|
||||
def fillScreen(self, c):
|
||||
self.fillRect(0, 0, self.width(), self.height(), c)
|
||||
|
||||
@classmethod
|
||||
def drawLine(self, x0, y0, x1, y1, c):
|
||||
self.startWrite()
|
||||
self.writeLine(x0, y0, x1, y1, c)
|
||||
self.endWrite()
|
||||
|
||||
@classmethod
|
||||
def drawRect(self, x, y, w, h, c):
|
||||
self.GFX.rect(x, y, w, h, c)
|
||||
|
||||
@classmethod
|
||||
def drawCircle(self, x, y, r, c):
|
||||
self.GFX.circle(x, y, r, c)
|
||||
|
||||
@classmethod
|
||||
def fillCircle(self, x, y, r, c):
|
||||
self.GFX.fill_circle(x, y, r, c)
|
||||
|
||||
@classmethod
|
||||
def drawTriangle(self, x0, y0, x1, y1, x2, y2, c):
|
||||
self.GFX.triangle(x0, y0, x1, y1, x2, y2, c)
|
||||
|
||||
@classmethod
|
||||
def fillTriangle(self, x0, y0, x1, y1, x2, y2, c):
|
||||
self.GFX.fill_triangle(x0, y0, x1, y1, x2, y2, c)
|
||||
|
||||
@classmethod
|
||||
def drawRoundRect(self, x, y, q, h, r, c):
|
||||
self.GFX.round_rect(x, y, q, h, r, c)
|
||||
|
||||
@classmethod
|
||||
def fillRoundRect(self, x, y, q, h, r, c):
|
||||
self.GFX.fill_round_rect(x, y, q, h, r, c)
|
||||
|
||||
@classmethod
|
||||
def setTextSize(self, s):
|
||||
self.textSize = s
|
||||
|
||||
@classmethod
|
||||
def setFont(self, f):
|
||||
self.GFX.font = f
|
||||
|
||||
@classmethod
|
||||
def printText(self, x, y, s, c=BLACK):
|
||||
self.GFX._very_slow_text(x, y, s, self.textSize, c)
|
||||
|
||||
@classmethod
|
||||
def drawBitmap(self, x, y, data, w, h, c=BLACK):
|
||||
byteWidth = (w + 7) // 8
|
||||
byte = 0
|
||||
self.startWrite()
|
||||
for j in range(h):
|
||||
for i in range(w):
|
||||
if i & 7:
|
||||
byte <<= 1
|
||||
else:
|
||||
byte = data[j * byteWidth + i // 8]
|
||||
if byte & 0x80:
|
||||
self.writePixel(x + i, y + j, c)
|
||||
self.endWrite()
|
File diff suppressed because it is too large
Load Diff
|
@ -3,11 +3,12 @@ import time
|
|||
import os
|
||||
from machine import ADC, I2C, SPI, Pin, SDCard
|
||||
from micropython import const
|
||||
from PCAL6416A import *
|
||||
from shapes import Shapes
|
||||
from mcp23017 import MCP23017
|
||||
from machine import Pin as mPin
|
||||
from gfx import GFX
|
||||
from gfx_standard_font_01 import text_dict as std_font
|
||||
|
||||
# ===== Constants that change between the Inkplate 6 and 10
|
||||
|
||||
# Connections between ESP32 and color Epaper
|
||||
|
@ -50,60 +51,24 @@ VCM_DC_SET_REGISTER = "\x02"
|
|||
D_COLS = const(600)
|
||||
D_ROWS = const(448)
|
||||
|
||||
MCP23017_INT_ADDR = const(0x20)
|
||||
MCP23017_EXT_ADDR = const(0x20)
|
||||
# User pins on PCAL6416A for Inkplate COLOR
|
||||
IO_PIN_A0 = const(0)
|
||||
IO_PIN_A1 = const(1)
|
||||
IO_PIN_A2 = const(2)
|
||||
IO_PIN_A3 = const(3)
|
||||
IO_PIN_A4 = const(4)
|
||||
IO_PIN_A5 = const(5)
|
||||
IO_PIN_A6 = const(6)
|
||||
IO_PIN_A7 = const(7)
|
||||
|
||||
MCP23017_INT_PORTA = const(0x00)
|
||||
MCP23017_INT_PORTB = const(0x01)
|
||||
MCP23017_INT_NO_MIRROR = False
|
||||
MCP23017_INT_MIRROR = True
|
||||
MCP23017_INT_PUSHPULL = False
|
||||
MCP23017_INT_OPENDRAIN = True
|
||||
MCP23017_INT_ACTLOW = False
|
||||
MCP23017_INT_ACTHIGH = True
|
||||
|
||||
MCP23017_IODIRA = const(0x00)
|
||||
MCP23017_IPOLA = const(0x02)
|
||||
MCP23017_GPINTENA = const(0x04)
|
||||
MCP23017_DEFVALA = const(0x06)
|
||||
MCP23017_INTCONA = const(0x08)
|
||||
MCP23017_IOCONA = const(0x0A)
|
||||
MCP23017_GPPUA = const(0x0C)
|
||||
MCP23017_INTFA = const(0x0E)
|
||||
MCP23017_INTCAPA = const(0x10)
|
||||
MCP23017_GPIOA = const(0x12)
|
||||
MCP23017_OLATA = const(0x14)
|
||||
|
||||
MCP23017_IODIRB = const(0x01)
|
||||
MCP23017_IPOLB = const(0x03)
|
||||
MCP23017_GPINTENB = const(0x05)
|
||||
MCP23017_DEFVALB = const(0x07)
|
||||
MCP23017_INTCONB = const(0x09)
|
||||
MCP23017_IOCONB = const(0x0B)
|
||||
MCP23017_GPPUB = const(0x0D)
|
||||
MCP23017_INTFB = const(0x0F)
|
||||
MCP23017_INTCAPB = const(0x11)
|
||||
MCP23017_GPIOB = const(0x13)
|
||||
MCP23017_OLATB = const(0x15)
|
||||
|
||||
# User pins on MCP for Inkplate COLOR
|
||||
MCP23017_PIN_A0 = const(0)
|
||||
MCP23017_PIN_A1 = const(1)
|
||||
MCP23017_PIN_A2 = const(2)
|
||||
MCP23017_PIN_A3 = const(3)
|
||||
MCP23017_PIN_A4 = const(4)
|
||||
MCP23017_PIN_A5 = const(5)
|
||||
MCP23017_PIN_A6 = const(6)
|
||||
MCP23017_PIN_A7 = const(7)
|
||||
|
||||
MCP23017_PIN_B0 = const(8)
|
||||
MCP23017_PIN_B1 = const(9)
|
||||
MCP23017_PIN_B2 = const(10)
|
||||
MCP23017_PIN_B3 = const(11)
|
||||
MCP23017_PIN_B4 = const(12)
|
||||
MCP23017_PIN_B5 = const(13)
|
||||
MCP23017_PIN_B6 = const(14)
|
||||
MCP23017_PIN_B7 = const(15)
|
||||
IO_PIN_B0 = const(8)
|
||||
IO_PIN_B1 = const(9)
|
||||
IO_PIN_B2 = const(10)
|
||||
IO_PIN_B3 = const(11)
|
||||
IO_PIN_B4 = const(12)
|
||||
IO_PIN_B5 = const(13)
|
||||
IO_PIN_B6 = const(14)
|
||||
IO_PIN_B7 = const(15)
|
||||
|
||||
|
||||
class Inkplate:
|
||||
|
@ -125,28 +90,10 @@ class Inkplate:
|
|||
|
||||
_framebuf = bytearray([0x11] * (D_COLS * D_ROWS // 2))
|
||||
|
||||
@classmethod
|
||||
def __init__(self):
|
||||
try:
|
||||
os.mount(
|
||||
SDCard(
|
||||
slot=3,
|
||||
miso=Pin(12),
|
||||
mosi=Pin(13),
|
||||
sck=Pin(14),
|
||||
cs=Pin(15)),
|
||||
"/sd"
|
||||
)
|
||||
except:
|
||||
print("Sd card could not be read")
|
||||
|
||||
@classmethod
|
||||
def begin(self):
|
||||
self.wire = I2C(0, scl=Pin(22), sda=Pin(21))
|
||||
self._mcp23017 = MCP23017(self.wire)
|
||||
self.TOUCH1 = self._mcp23017.pin(10, Pin.IN)
|
||||
self.TOUCH2 = self._mcp23017.pin(11, Pin.IN)
|
||||
self.TOUCH3 = self._mcp23017.pin(12, Pin.IN)
|
||||
self._PCAL6416A = PCAL6416A(self.wire)
|
||||
|
||||
self.spi = SPI(2)
|
||||
|
||||
|
@ -157,6 +104,8 @@ class Inkplate:
|
|||
self.EPAPER_DC_PIN = Pin(EPAPER_DC_PIN, Pin.OUT)
|
||||
self.EPAPER_CS_PIN = Pin(EPAPER_CS_PIN, Pin.OUT)
|
||||
|
||||
self.SD_ENABLE = gpioPin(self._PCAL6416A, 10, modeOUTPUT)
|
||||
|
||||
self.framebuf = bytearray(D_ROWS * D_COLS // 2)
|
||||
|
||||
self.GFX = GFX(
|
||||
|
@ -205,25 +154,41 @@ class Inkplate:
|
|||
self.sendCommand(b"\x50")
|
||||
self.sendData(b"\x37")
|
||||
|
||||
self.setMCPForLowPower()
|
||||
self.setPCALForLowPower()
|
||||
|
||||
self._panelState = True
|
||||
|
||||
return True
|
||||
|
||||
def initSDCard(self):
|
||||
self.SD_ENABLE.digitalWrite(0)
|
||||
try:
|
||||
os.mount(
|
||||
SDCard(
|
||||
slot=3,
|
||||
miso=Pin(12),
|
||||
mosi=Pin(13),
|
||||
sck=Pin(14),
|
||||
cs=Pin(15)),
|
||||
"/sd"
|
||||
)
|
||||
except:
|
||||
print("Sd card could not be read")
|
||||
|
||||
def SDCardSleep(self):
|
||||
self.SD_ENABLE.digitalWrite(1)
|
||||
time.sleep_ms(5)
|
||||
|
||||
def SDCardWake(self):
|
||||
self.SD_ENABLE.digitalWrite(0)
|
||||
time.sleep_ms(5)
|
||||
|
||||
@classmethod
|
||||
def setMCPForLowPower(self):
|
||||
self._mcp23017.pin(10, mode=mPin.IN)
|
||||
self._mcp23017.pin(11, mode=mPin.IN)
|
||||
self._mcp23017.pin(12, mode=mPin.IN)
|
||||
def setPCALForLowPower(self):
|
||||
|
||||
self._mcp23017.pin(9, value=0, mode=mPin.OUT)
|
||||
|
||||
for x in range(8):
|
||||
self._mcp23017.pin(x, value=0, mode=mPin.OUT)
|
||||
self._mcp23017.pin(8, value=0, mode=mPin.OUT)
|
||||
self._mcp23017.pin(13, value=0, mode=mPin.OUT)
|
||||
self._mcp23017.pin(14, value=0, mode=mPin.OUT)
|
||||
self._mcp23017.pin(15, value=0, mode=mPin.OUT)
|
||||
for x in range(16):
|
||||
self._PCAL6416A.pinMode(int(x), modeOUTPUT)
|
||||
self._PCAL6416A.digitalWrite(int(x), 0)
|
||||
|
||||
@classmethod
|
||||
def getPanelDeepSleepState(self):
|
||||
|
@ -304,6 +269,10 @@ class Inkplate:
|
|||
|
||||
time.sleep_ms(200)
|
||||
|
||||
@classmethod
|
||||
def gpioExpanderPin(self, pin, mode):
|
||||
return gpioPin(self._PCAL6416A, pin, mode)
|
||||
|
||||
@classmethod
|
||||
def clean(self):
|
||||
if not self._panelState:
|
||||
|
@ -386,8 +355,8 @@ class Inkplate:
|
|||
_x_sub = x % 2
|
||||
|
||||
temp = self._framebuf[D_COLS * y // 2 + _x]
|
||||
self._framebuf[D_COLS * y // 2 + _x] = (pixelMaskGLUT[_x_sub] & temp) |\
|
||||
(c if _x_sub else c << 4)
|
||||
self._framebuf[D_COLS * y // 2 + _x] = (pixelMaskGLUT[_x_sub] & temp) | \
|
||||
(c if _x_sub else c << 4)
|
||||
|
||||
@classmethod
|
||||
def writeFillRect(self, x, y, w, h, c):
|
||||
|
@ -517,103 +486,3 @@ class Inkplate:
|
|||
if byte & 0x80:
|
||||
self.writePixel(x + i, y + j, c)
|
||||
self.endWrite()
|
||||
|
||||
# @classmethod
|
||||
# def drawImageFile(self, x, y, path, invert=False):
|
||||
# with open(path, "rb") as f:
|
||||
# header14 = f.read(14)
|
||||
# if header14[0] != 0x42 or header14[1] != 0x4D:
|
||||
# return 0
|
||||
# header40 = f.read(40)
|
||||
|
||||
# w = int(
|
||||
# (header40[7] << 24)
|
||||
# + (header40[6] << 16)
|
||||
# + (header40[5] << 8)
|
||||
# + header40[4]
|
||||
# )
|
||||
# h = int(
|
||||
# (header40[11] << 24)
|
||||
# + (header40[10] << 16)
|
||||
# + (header40[9] << 8)
|
||||
# + header40[8]
|
||||
# )
|
||||
# dataStart = int((header14[11] << 8) + header14[10])
|
||||
|
||||
# depth = int((header40[15] << 8) + header40[14])
|
||||
# totalColors = int((header40[33] << 8) + header40[32])
|
||||
|
||||
# rowSize = 4 * ((depth * w + 31) // 32)
|
||||
|
||||
# if totalColors == 0:
|
||||
# totalColors = 1 << depth
|
||||
|
||||
# palette = None
|
||||
|
||||
# if depth <= 8:
|
||||
# palette = [0 for i in range(totalColors)]
|
||||
# p = f.read(totalColors * 4)
|
||||
# for i in range(totalColors):
|
||||
# palette[i] = (
|
||||
# 54 * p[i * 4] + 183 * p[i * 4 + 1] + 19 * p[i * 4 + 2]
|
||||
# ) >> 14
|
||||
# # print(palette)
|
||||
# f.seek(dataStart)
|
||||
# for j in range(h):
|
||||
# # print(100 * j // h, "% complete")
|
||||
# buffer = f.read(rowSize)
|
||||
# for i in range(w):
|
||||
# val = 0
|
||||
# if depth == 1:
|
||||
# px = int(
|
||||
# invert
|
||||
# ^ (palette[0] < palette[1])
|
||||
# ^ bool(buffer[i >> 3] & (1 << (7 - i & 7)))
|
||||
# )
|
||||
# val = palette[px]
|
||||
# elif depth == 4:
|
||||
# px = (buffer[i >> 1] & (0x0F if i & 1 == 1 else 0xF0)) >> (
|
||||
# 0 if i & 1 else 4
|
||||
# )
|
||||
# val = palette[px]
|
||||
# if invert:
|
||||
# val = 3 - val
|
||||
# elif depth == 8:
|
||||
# px = buffer[i]
|
||||
# val = palette[px]
|
||||
# if invert:
|
||||
# val = 3 - val
|
||||
# elif depth == 16:
|
||||
# px = (buffer[(i << 1) | 1] << 8) | buffer[(i << 1)]
|
||||
|
||||
# r = (px & 0x7C00) >> 7
|
||||
# g = (px & 0x3E0) >> 2
|
||||
# b = (px & 0x1F) << 3
|
||||
|
||||
# val = (54 * r + 183 * g + 19 * b) >> 14
|
||||
|
||||
# if invert:
|
||||
# val = 3 - val
|
||||
# elif depth == 24:
|
||||
# r = buffer[i * 3]
|
||||
# g = buffer[i * 3 + 1]
|
||||
# b = buffer[i * 3 + 2]
|
||||
|
||||
# val = (54 * r + 183 * g + 19 * b) >> 14
|
||||
|
||||
# if invert:
|
||||
# val = 3 - val
|
||||
# elif depth == 32:
|
||||
# r = buffer[i * 4]
|
||||
# g = buffer[i * 4 + 1]
|
||||
# b = buffer[i * 4 + 2]
|
||||
|
||||
# val = (54 * r + 183 * g + 19 * b) >> 14
|
||||
|
||||
# if invert:
|
||||
# val = 3 - val
|
||||
|
||||
# if self.getDisplayMode() == self.INKPLATE_1BIT:
|
||||
# val >>= 1
|
||||
|
||||
# self.drawPixel(x + i, y + h - j, val)
|
||||
|
|
File diff suppressed because it is too large
Load Diff
File diff suppressed because it is too large
Load Diff
File diff suppressed because it is too large
Load Diff
|
@ -0,0 +1,3 @@
|
|||
soldered_logo = bytearray(
|
||||
b"\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\xfc\x3f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\xf0\x0f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\xc0\x07\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\x80\x01\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xfe\x00\x00\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xf8\x00\x00\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xf0\x00\x00\x01\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xc0\x01\x80\x01\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xc0\x07\xe0\x00\xff\xfe\x03\xff\xf8\x07\xfe\x07\xff\xc0\x0f\xff\x80\x00\x78\x00\xff\xf0\x00\x1e\x00\x7f\xe0\xc0\x1f\xf0\x00\xff\xf8\x00\xff\xe0\x01\xfe\x07\xff\xc0\x00\xff\x80\x00\x78\x00\x0f\xf0\x00\x0e\x00\x0f\xe0\xc0\x3f\xfc\x00\xff\xf0\x00\x3f\x80\x00\xfe\x07\xff\xc0\x00\x3f\x80\x00\x78\x00\x03\xf0\x00\x0e\x00\x03\xe0\xc0\xfe\x7e\x00\xff\xe0\x00\x3f\x00\x00\x7e\x07\xff\xc0\x00\x1f\x80\x00\x78\x00\x01\xf0\x00\x0e\x00\x00\xe0\xc0\xfc\x3f\x01\xff\xc0\x00\x3f\x00\x00\x3e\x07\xff\xc0\x00\x0f\x80\x00\x78\x00\x01\xf0\x00\x0e\x00\x00\x60\xc0\xf8\x0f\x81\xff\xc0\x00\x7e\x00\x80\x1e\x07\xff\xc0\x00\x0f\x80\x00\x78\x1c\x00\xf0\x00\x1e\x00\x00\x60\xc0\xf8\x03\xff\xff\x80\xfc\xfe\x03\xf0\x1e\x07\xff\xc0\xf8\x07\x81\xff\xf8\x1f\x80\xf0\x3f\xfe\x07\xc0\x20\xc0\xf8\x01\xff\xff\x80\xff\xfc\x07\xf8\x0e\x07\xff\xc0\xfc\x07\x81\xff\xf8\x1f\xc0\xf0\x3f\xfe\x07\xe0\x20\xc0\xf8\x00\x7f\xff\x80\xff\xfc\x0f\xf8\x0e\x07\xff\xc0\xfe\x03\x81\xff\xf8\x1f\xc0\xf0\x3f\xfe\x07\xf0\x00\xc0\xfc\x00\x1f\xff\x80\x7f\xfc\x0f\xfc\x0e\x07\xff\xc0\xfe\x03\x81\xff\xf8\x1f\xc0\xf0\x3f\xfe\x07\xf0\x00\xc0\x7e\x00\x07\xff\xc0\x0f\xf8\x0f\xfc\x0e\x07\xff\xc0\xff\x03\x80\x00\xf8\x1f\x80\xf0\x00\x3e\x07\xf0\x00\xc0\x1f\x80\x03\xff\xc0\x03\xf8\x0f\xfc\x0e\x07\xff\xc0\xff\x03\x80\x00\xf8\x1f\x00\xf0\x00\x3e\x07\xf8\x00\xc0\x0f\xc0\x01\xff\xe0\x00\xf8\x0f\xfc\x0e\x07\xff\xc0\xff\x03\x80\x00\xf8\x00\x01\xf0\x00\x3e\x07\xf8\x00\xc0\x03\xf0\x01\xff\xf0\x00\x78\x0f\xfc\x0e\x07\xff\xc0\xff\x03\x80\x00\xf8\x00\x01\xf0\x00\x3e\x07\xf8\x00\xc0\x00\xfc\x01\xff\xfc\x00\x38\x0f\xfc\x0e\x07\xff\xc0\xff\x03\x80\x00\xf8\x00\x03\xf0\x00\x3e\x07\xf8\x00\xf0\x00\x3f\x01\xff\xff\x00\x18\x0f\xfc\x0e\x07\xff\xc0\xff\x03\x80\x01\xf8\x00\x0f\xf0\x00\x3e\x07\xf8\x00\xfc\x00\x1f\x01\xff\xff\xc0\x1c\x0f\xfc\x0e\x07\xff\xc0\xff\x03\x81\xff\xf8\x00\x0f\xf0\x3f\xfe\x07\xf0\x00\xfe\x00\x1f\x01\xff\xff\xf0\x1c\x0f\xf8\x0e\x03\xff\xc0\xfe\x03\x81\xff\xf8\x1c\x07\xf0\x3f\xfe\x07\xf0\x00\xff\x80\x1f\x01\xff\xff\xf8\x1c\x07\xf8\x0e\x01\xff\xc0\xfe\x07\x81\xff\xf8\x1c\x07\xf0\x3f\xfe\x07\xe0\x20\xf3\xe0\x1f\x01\xff\xe7\xf8\x1c\x03\xf0\x1e\x00\xff\xc0\xfc\x07\x81\xff\xf8\x1e\x03\xf0\x3f\xfe\x07\xe0\x20\xc0\xf8\x1f\x01\xff\xc1\xf0\x1e\x01\xe0\x1f\x00\x01\xc0\xf0\x07\x81\xff\xf8\x1e\x03\xf0\x3f\xfe\x07\x80\x60\x80\xfc\x1f\x01\xff\x80\x00\x1e\x00\x00\x3f\x80\x01\xc0\x00\x0f\x80\x00\x38\x1f\x01\xf0\x00\x0e\x00\x00\x60\x80\x7f\x7f\x01\xff\x80\x00\x3f\x00\x00\x3f\xc0\x01\xc0\x00\x1f\x80\x00\x38\x1f\x01\xf0\x00\x0e\x00\x00\xe0\x00\x1f\xfc\x01\xff\x80\x00\x3f\x80\x00\x7f\xe0\x01\xc0\x00\x3f\x80\x00\x38\x1f\x80\xf0\x00\x0e\x00\x01\xe0\x00\x0f\xf8\x01\xff\xc0\x00\xff\xc0\x00\xff\xf0\x01\xc0\x00\x7f\x80\x00\x38\x1f\x80\xf0\x00\x0e\x00\x03\xe0\x80\x03\xe0\x01\xff\xf0\x01\xff\xf0\x03\xff\xf8\x01\xc0\x03\xff\x80\x00\x38\x1f\xc0\x70\x00\x0e\x00\x1f\xe0\x80\x00\x80\x03\xff\xff\x1f\xff\xfe\x3f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xc0\x00\x00\x07\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xfc\x00\x00\x1f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\x00\x00\x7f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\xc0\x00\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\xe0\x03\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\xf8\x0f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\xfe\x3f\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xff\xe0"
|
||||
)
|
Loading…
Reference in New Issue